用vue做一个轮播图页面
时间: 2023-08-30 19:12:39 浏览: 140
好的,以下是用 Vue 实现一个简单的轮播图页面的步骤:
1. 创建一个 Vue 组件,命名为 `Slider`。
2. 在 `Slider` 组件中定义数据,包括轮播图的图片列表、当前图片的索引、定时器等。
```javascript
data() {
return {
images: [
'https://example.com/image1.jpg',
'https://example.com/image2.jpg',
'https://example.com/image3.jpg'
],
currentIndex: 0,
timer: null
}
}
```
3. 在 `Slider` 组件中编写模板,包括轮播图区域和下方的小圆点。
```html
<template>
<div class="slider">
<div class="slider-container">
<img v-for="(image, index) in images" :src="image" :key="index" :class="{ active: currentIndex === index }">
</div>
<div class="slider-dots">
<span v-for="(image, index) in images" :key="index" :class="{ active: currentIndex === index }" @click="jumpTo(index)"></span>
</div>
</div>
</template>
```
4. 在 `Slider` 组件中编写样式,包括轮播图区域和小圆点的样式。
```css
.slider {
position: relative;
width: 100%;
height: 500px;
}
.slider-container {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
overflow: hidden;
}
.slider-container img {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
opacity: 0;
transition: opacity 0.5s ease-in-out;
}
.slider-container img.active {
opacity: 1;
}
.slider-dots {
position: absolute;
bottom: 20px;
left: 50%;
transform: translateX(-50%);
}
.slider-dots span {
display: inline-block;
width: 10px;
height: 10px;
border-radius: 50%;
background-color: #ccc;
margin: 0 5px;
cursor: pointer;
}
.slider-dots span.active {
background-color: #f00;
}
```
5. 在 `Slider` 组件中编写方法,实现轮播图的自动播放和手动切换。
```javascript
methods: {
startAutoPlay() {
this.timer = setInterval(() => {
this.currentIndex = (this.currentIndex + 1) % this.images.length;
}, 2000);
},
stopAutoPlay() {
clearInterval(this.timer);
},
jumpTo(index) {
this.currentIndex = index;
}
},
mounted() {
this.startAutoPlay();
},
beforeDestroy() {
this.stopAutoPlay();
}
```
6. 在父组件中使用 `Slider` 组件,并传入必要的属性。
```html
<template>
<div>
<slider :images="['https://example.com/image1.jpg', 'https://example.com/image2.jpg', 'https://example.com/image3.jpg']"></slider>
</div>
</template>
```
7. 在应用程序中引入必要的 CSS 文件和 Vue 库文件。
```html
<head>
<link rel="stylesheet" href="path/to/slider.css">
</head>
<body>
<div id="app">
<!-- 在这里使用父组件 -->
</div>
<script src="path/to/vue.js"></script>
</body>
```
这样就完成了一个简单的轮播图页面的实现。需要注意的是,这只是一个基础的实现,还可以根据需求进行扩展,比如添加动画效果、优化性能等。
阅读全文
相关推荐
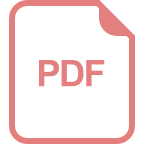
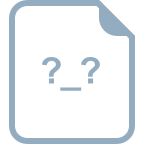

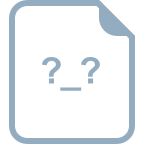
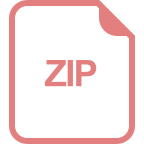
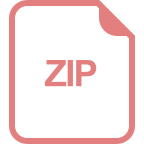
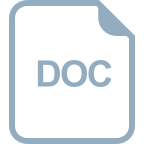









