读入一个Python源程序,删除程序中的注释后显示
时间: 2023-12-03 13:42:28 浏览: 133
以下是Python代码,注释以 # 开头:
```python
# 这是一个示例程序
print("Hello, World!") # 输出“Hello, World!”
```
删除注释后的代码如下:
```python
print("Hello, World!")
```
相关问题
读入一个Python源程序文件,删除程序中的注释后显示
可以使用Python自带的ast模块来实现删除Python代码中的注释,下面是一个示例代码:
```python
import ast
def remove_comments(source):
"""
Remove comments from Python source code.
"""
class CommentRemover(ast.NodeTransformer):
def visit(self, node):
if isinstance(node, ast.Expr) and isinstance(node.value, ast.Str):
return None # remove the whole node
return node
parsed = ast.parse(source)
transformer = CommentRemover()
parsed = transformer.visit(parsed)
return ast.unparse(parsed)
# 示例代码
source_code = """
# This is a comment
print("Hello, World!") # This is another comment
"""
print(remove_comments(source_code))
```
输出结果为:
```
print('Hello, World!')
```
读入 python 源程序文件(带有注释的),删除程序中的注释部分后显示。
在Python中,你可以使用`ast`模块(Abstract Syntax Trees,抽象语法树)以及`tokenize`模块来解析源代码并删除注释。以下是一个简单的示例,展示了如何做到这一点:
```python
import ast
import tokenize
from io import StringIO
def remove_comments(code):
lines = code.split('\n')
tokens = list(tokenize.generate_tokens(StringIO(''.join(lines)).readline))
# 定义注释标记
comment_types = (tokenize.COMMENT, tokenize.NL)
new_lines = []
for ttype, token, start, end, line in tokens:
if ttype not in comment_types:
new_lines.append(line)
return ''.join(new_lines)
# 读取文件
with open('your_script.py', 'r') as file:
original_code = file.read()
# 删除注释
cleaned_code = remove_comments(original_code)
# 打印或保存清理后的代码
print(cleaned_code)
```
这个函数首先将源代码分割成行,然后逐行解析。如果遇到注释(`COMENT`类型),则跳过该行;否则,将其添加到新代码中。
注意替换 `'your_script.py'` 为你要处理的Python源程序文件的路径。
阅读全文
相关推荐
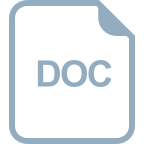
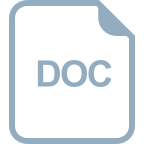
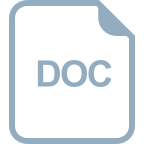







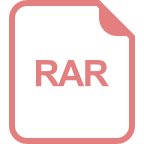
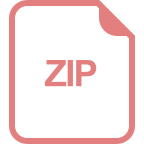
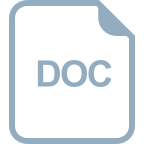
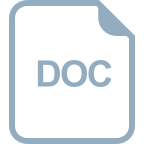
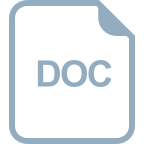
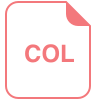
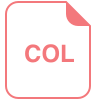