在Unity中写两个方法,分别用来保存和读取下面这些数据 public string savePath; public string serverPath; public BuildAssetBundleOptions bundleOptions; public BuildTarget buildTarget; public bool copyToServerPath;
时间: 2023-05-28 09:07:07 浏览: 93
以下是在Unity中保存和读取数据的两个方法:
```csharp
using UnityEngine;
using System.IO;
using System.Runtime.Serialization.Formatters.Binary;
public class DataManager : MonoBehaviour
{
public string savePath;
public string serverPath;
public BuildAssetBundleOptions bundleOptions;
public BuildTarget buildTarget;
public bool copyToServerPath;
// 保存数据到本地
public void SaveData()
{
BinaryFormatter formatter = new BinaryFormatter();
string path = Application.persistentDataPath + "/" + savePath;
FileStream stream = new FileStream(path, FileMode.Create);
DataToSave data = new DataToSave(savePath, serverPath, bundleOptions, buildTarget, copyToServerPath);
formatter.Serialize(stream, data);
stream.Close();
}
// 从本地读取数据
public void LoadData()
{
string path = Application.persistentDataPath + "/" + savePath;
if (File.Exists(path))
{
BinaryFormatter formatter = new BinaryFormatter();
FileStream stream = new FileStream(path, FileMode.Open);
DataToSave data = formatter.Deserialize(stream) as DataToSave;
stream.Close();
savePath = data.savePath;
serverPath = data.serverPath;
bundleOptions = data.bundleOptions;
buildTarget = data.buildTarget;
copyToServerPath = data.copyToServerPath;
}
else
{
Debug.Log("Save file not found in " + path);
}
}
}
[System.Serializable]
public class DataToSave
{
public string savePath;
public string serverPath;
public BuildAssetBundleOptions bundleOptions;
public BuildTarget buildTarget;
public bool copyToServerPath;
public DataToSave(string savePath, string serverPath, BuildAssetBundleOptions bundleOptions, BuildTarget buildTarget, bool copyToServerPath)
{
this.savePath = savePath;
this.serverPath = serverPath;
this.bundleOptions = bundleOptions;
this.buildTarget = buildTarget;
this.copyToServerPath = copyToServerPath;
}
}
```
这个类包含了两个方法:SaveData()和LoadData()。SaveData()方法将数据保存到本地,LoadData()方法从本地读取数据。数据以二进制格式序列化并保存在应用程序的持久数据路径中。在LoadData()方法中,我们反序列化数据并将其存储在类的变量中。
在这个示例中,我们将数据存储在一个名为DataToSave的类中,该类包含了所有要保存的变量。我们使用BinaryFormatter将这个类序列化为二进制格式并保存到本地。在LoadData()方法中,我们使用相同的BinaryFormatter反序列化数据并将其存储在我们的变量中。
阅读全文
相关推荐
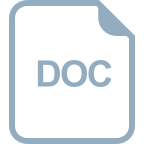
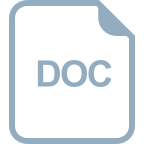
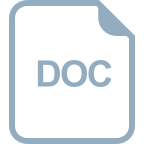

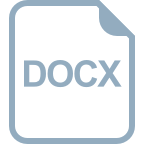
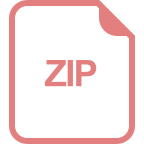
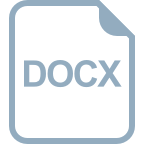
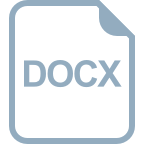
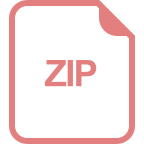
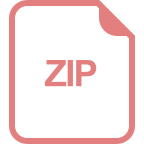
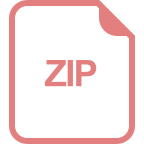
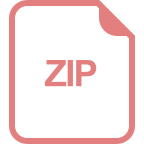
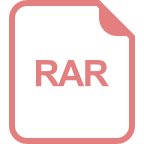
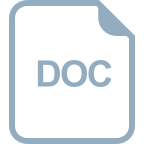