ffmpeg打开rtsp
时间: 2024-07-05 11:01:22 浏览: 150
FFmpeg是一个强大的跨平台多媒体处理工具,它可以用来处理各种流媒体协议,包括RTSP(Real Time Streaming Protocol)。要使用FFmpeg打开RTSP流,你可以使用`ffplay`命令行工具,它是FFmpeg的一部分,专门用于播放实时或非实时数据。
基本的命令格式如下:
```sh
ffplay [options] rtsp://[server_url]:[port]/[stream_path]
```
例如,如果你想要播放URL `rtsp://your-server.com/live/stream`,命令会是:
```sh
ffplay rtsp://your-server.com/live/stream
```
这里,`server_url`是你RTSP服务器的地址,`port`通常为554(对于H.264和H.265),`stream_path`是视频流的路径。你可以添加其他选项来自定义播放行为,如调整音视频质量、音频轨道选择等。
相关问题:
1. FFmpeg的`ffplay`除了播放RTSP,还能用来做什么?
2. 如何查看RTSP流的详细信息以便更好地设置`ffplay`参数?
3. 如果RTSP流需要身份验证,如何在命令中添加凭证?
相关问题
用ffmpeg打开RTSP流,并依次传输不同的RTSP流,并在接收端分开接收并保存在本地,C代码
在C语言中,你可以使用FFmpeg库来处理RTSP流。以下是一个简单的示例,展示了如何使用FFmpeg打开RTSP流,同时接收并保存多个流到本地文件:
```c
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libavutil/avutil.h>
// 函数原型声明
int open_rtsp(const char *url, AVFormatContext **fmt_ctx);
void save_stream_to_file(AVStream *stream, const char *filename);
int main() {
// 创建一个AVFormatContext结构体指针
AVFormatContext *fmt_ctx = NULL;
// 这里假设你有一个包含多个RTSP地址的数组
const char *rtsp_urls[] = {"rtsp://example.com/stream1", "rtsp://example.com/stream2", "rtsp://example.com/stream3"};
int num_streams = sizeof(rtsp_urls) / sizeof(rtsp_urls[0]);
for (int i = 0; i < num_streams; i++) {
if (open_rtsp(rtsp_urls[i], &fmt_ctx)) {
printf("Failed to open RTSP stream %d\n", i + 1);
continue;
}
// 遍历所有视频流
for (int j = 0; j < fmt_ctx->nb_streams; j++) {
AVStream *stream = fmt_ctx->streams[j];
if (stream->codec->codec_type == AVMEDIA_TYPE_VIDEO) {
char filename[128]; // 文件名格式化字符串
snprintf(filename, sizeof(filename), "output%d_%s.mp4", i + 1, stream-> codec->name); // 根据流编号和编码器命名文件
save_stream_to_file(stream, filename);
avformat_close_input(&fmt_ctx);
break; // 只保存第一个视频流
}
}
}
return 0;
}
// 打开RTSP流函数
int open_rtsp(const char *url, AVFormatContext **fmt_ctx) {
*fmt_ctx = NULL;
if (!avformat_open_input(fmt_ctx, url, NULL, NULL)) {
fprintf(stderr, "Error opening RTSP stream: %s\n", url);
return -1;
}
if (!avformat_find_stream_info(*fmt_ctx, NULL)) {
fprintf(stderr, "Error finding stream info: %s\n", url);
return -1;
}
return 0;
}
// 将流保存到文件函数
void save_stream_to_file(AVStream *stream, const char *filename) {
FILE *out = fopen(filename, "wb");
if (!out) {
perror("Failed to open output file");
return;
}
AVCodecContext *codec_ctx = stream->codec;
AVIOContext *pb = avio_alloc_context(out);
AVPacket pkt;
while (1) {
av_read_frame(stream->pb, &pkt);
if (pkt.stream_index == stream->index && pkt.data) {
avcodec_send_packet(codec_ctx, &pkt);
while (avcodec_receive_packet(codec_ctx, &pkt) >= 0) {
av_interleaved_write_frame(pb, &pkt);
}
}
av_free_packet(&pkt);
if (pkt.stream_index != stream->index || !pkt.data)
break;
}
fclose(out);
}
```
注意:这个示例假设你已经包含了FFmpeg库并且正确安装了它。在实际项目中,你可能需要处理更多的错误情况,并使用线程安全的方式来并发处理多个流。
ffmpeg 解码rtsp
可以使用 ffmpeg 库来解码 RTSP 视频流。下面是一个简单的 C++ 代码示例,使用 ffmpeg 库解码 RTSP 视频流:
```cpp
#include <iostream>
#include <thread>
#include <chrono>
extern "C" {
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libswscale/swscale.h>
}
int main(int argc, char *argv[]) {
AVFormatContext *pFormatCtx = nullptr;
AVCodecContext *pCodecCtxOrig = nullptr;
AVCodecContext *pCodecCtx = nullptr;
AVCodec *pCodec = nullptr;
AVFrame *pFrame = nullptr;
AVPacket *packet = nullptr;
// RTSP URL
const char *url = "rtsp://example.com/stream";
// Register all formats and codecs
av_register_all();
avformat_network_init();
// Open RTSP stream
if (avformat_open_input(&pFormatCtx, url, nullptr, nullptr) != 0) {
std::cerr << "Could not open input stream" << std::endl;
return -1;
}
// Retrieve stream information
if (avformat_find_stream_info(pFormatCtx, nullptr) < 0) {
std::cerr << "Could not find stream information" << std::endl;
return -1;
}
// Find the first video stream
int videoStreamIndex = -1;
for (int i = 0; i < pFormatCtx->nb_streams; i++) {
if (pFormatCtx->streams[i]->codecpar->codec_type == AVMEDIA_TYPE_VIDEO) {
videoStreamIndex = i;
break;
}
}
// Check if video stream was found
if (videoStreamIndex == -1) {
std::cerr << "Could not find video stream" << std::endl;
return -1;
}
// Get a pointer to the codec context for the video stream
pCodecCtxOrig = pFormatCtx->streams[videoStreamIndex]->codec;
// Find the decoder for the video stream codec
pCodec = avcodec_find_decoder(pCodecCtxOrig->codec_id);
if (!pCodec) {
std::cerr << "Unsupported codec" << std::endl;
return -1;
}
// Copy context
pCodecCtx = avcodec_alloc_context3(pCodec);
if (avcodec_copy_context(pCodecCtx, pCodecCtxOrig) != 0) {
std::cerr << "Could not copy codec context" << std::endl;
return -1;
}
// Open codec
if (avcodec_open2(pCodecCtx, pCodec, nullptr) < 0) {
std::cerr << "Could not open codec" << std::endl;
return -1;
}
// Allocate video frame
pFrame = av_frame_alloc();
// Allocate packet
packet = av_packet_alloc();
// Read frames from the stream
while (av_read_frame(pFormatCtx, packet) >= 0) {
// Check if packet belongs to video stream
if (packet->stream_index == videoStreamIndex) {
// Send packet to decoder
avcodec_send_packet(pCodecCtx, packet);
// Receive decoded frame
int ret = avcodec_receive_frame(pCodecCtx, pFrame);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF) {
// Not enough data yet, or end of file
continue;
} else if (ret < 0) {
std::cerr << "Error decoding frame" << std::endl;
break;
}
// Convert frame to RGB
SwsContext *sws_ctx = sws_getContext(pCodecCtx->width, pCodecCtx->height, pCodecCtx->pix_fmt,
pCodecCtx->width, pCodecCtx->height, AV_PIX_FMT_RGB24,
SWS_BILINEAR, nullptr, nullptr, nullptr);
if (!sws_ctx) {
std::cerr << "Could not initialize the conversion context" << std::endl;
break;
}
AVFrame *rgbFrame = av_frame_alloc();
if (!rgbFrame) {
std::cerr << "Could not allocate RGB frame" << std::endl;
break;
}
av_image_alloc(rgbFrame->data, rgbFrame->linesize, pCodecCtx->width, pCodecCtx->height, AV_PIX_FMT_RGB24, 1);
sws_scale(sws_ctx, (const uint8_t *const *) pFrame->data, pFrame->linesize, 0, pCodecCtx->height,
rgbFrame->data, rgbFrame->linesize);
// Display frame
std::cout << "Frame width: " << pCodecCtx->width << ", height: " << pCodecCtx->height << std::endl;
// Free RGB frame
av_freep(&rgbFrame->data[0]);
av_frame_free(&rgbFrame);
sws_freeContext(sws_ctx);
}
// Free packet
av_packet_unref(packet);
// Sleep for a short time to simulate real-time playback
std::this_thread::sleep_for(std::chrono::milliseconds(33));
}
// Free resources
av_packet_free(&packet);
av_frame_free(&pFrame);
avcodec_close(pCodecCtx);
avcodec_free_context(&pCodecCtx);
avformat_close_input(&pFormatCtx);
avformat_network_deinit();
return 0;
}
```
这个代码示例使用了 ffmpeg 库中的 AVFormatContext、AVCodecContext、AVCodec、AVFrame 和 AVPacket 等数据类型,以及相关的函数来打开 RTSP 视频流,解码视频帧,并展示出来。这个代码示例中,我们将解码出来的视频帧转换为 RGB 格式,并输出帧的宽度和高度。你可以根据自己的需求来修改这个代码示例。
阅读全文
相关推荐
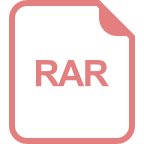
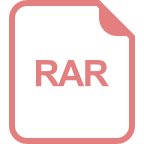
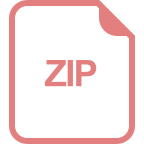
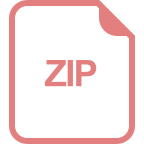
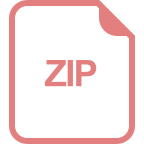




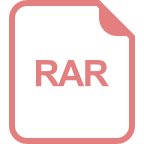






