编写函数判断某年数是否为闰年,主函数调用并输出
时间: 2023-05-11 13:05:46 浏览: 94
好的,以下是判断闰年的函数代码:
```python
def is_leap_year(year):
if year % 4 == 0 and year % 100 != 0 or year % 400 == 0:
return True
else:
return False
```
主函数调用并输出:
```python
year = int(input("请输入一个年份:"))
if is_leap_year(year):
print(year, "是闰年")
else:
print(year, "不是闰年")
```
请问还有什么问题需要我回答吗?
相关问题
c语言编写一个函数用于判断某年是否为闰年在主函数中调用此函数,判断用户输出的月份的天数
以下是C语言编写一个函数用于判断某年是否为闰年并在主函数中调用此函数,判断用户输出的月份的天数的代码:
```c
#include <stdio.h>
// 判断是否为闰年
int isLeapYear(int year) {
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) {
return 1;
} else {
return 0;
}
}
// 获取某年某月的天数
int getDays(int year, int month) {
int days;
switch (month) {
case 1:
case 3:
case 5:
case 7:
case 8:
case 10:
case 12:
days = 31;
break;
case 4:
case 6:
case 9:
case 11:
days = 30;
break;
case 2:
if (isLeapYear(year)) {
days = 29; } else {
days = 28;
}
break;
default:
days = -1;
break;
}
return days;
}
int main() {
int year, month;
printf("请输入年份和月份(如2022 2):");
scanf("%d %d", &year, &month);
int days = getDays(year, month);
if (days == -1) {
printf("输入的月份有误!\n");
} else {
printf("%d年%d月有%d天。\n", year, month, days);
}
return 0;
}
```
求某年某月的天数,比如2023年5月是31天。 要求:编写自定义函数实现相应功能,每个函数设计合适的参数和返回值: 1.编写函数isleapyear,判断某年是否是闰年 2.编写函数getdays,功能是对于任意年、月,获取该年该月的天数。 3.编写主函数main,输入年(year)、月(month),调用上述函数,得到该年该月的天数,输出天数。 【输入描述】共一行。有两个整数,第一个是年份,第二个是月份。年份和月份之间以一个空格隔开。 【输出描述】输出该年该月的天数。 【输入示例1】2017 11 【输出示例1】30 【输入示例2】2020 2 【输出示例2】29
```python
def isleapyear(year):
if (year % 4 == 0 and year % 100 != 0) or year % 400 == 0:
return True
else:
return False
def getdays(year, month):
days = [31,28,31,30,31,30,31,31,30,31,30,31] # 每月天数,2月先暂定为28天
if isleapyear(year): # 如果是闰年,2月改为29天
days[1] = 29
return days[month-1] # 返回对应月份的天数
def main():
year, month = map(int, input().split()) # 输入年份和月份
days = getdays(year, month) # 调用getdays函数获取该年该月的天数
print(days) # 输出天数
main()
```
阅读全文
相关推荐


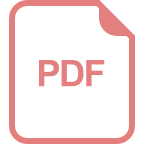
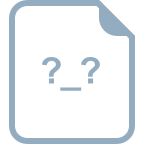
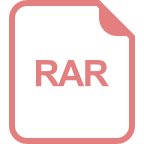
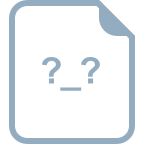
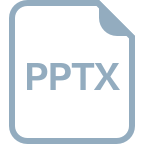
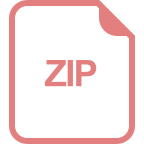
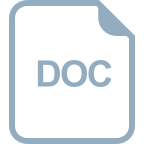




