用c++编写: 用类的组合技术,设计一个基本信息类、一个学生类、一个教师类。基本信息类包括姓名、性别、手机号、班级;学生类包括姓名、性别、手机号、班级、数学、语文和英语等成绩;教师类包括姓名、性别、手机号、班级、专业、教龄等。以上三个类必须包含构造函数,其他成员函数自行设计。主函数中,分别生成一个学生类和教师类对象,并调用成员函数。
时间: 2023-07-04 21:18:05 浏览: 79
好的,以下是用C++编写的程序:
```cpp
#include <iostream>
#include <string>
using namespace std;
// 基本信息类
class BasicInfo {
public:
BasicInfo(string name, string gender, string phone, string cls) :
name(name), gender(gender), phone(phone), cls(cls) {}
string getName() const { return name; }
string getGender() const { return gender; }
string getPhone() const { return phone; }
string getCls() const { return cls; }
private:
string name;
string gender;
string phone;
string cls;
};
// 学生类
class Student {
public:
Student(string name, string gender, string phone, string cls,
int math, int chinese, int english) :
info(name, gender, phone, cls), math(math), chinese(chinese), english(english) {}
string getName() const { return info.getName(); }
string getGender() const { return info.getGender(); }
string getPhone() const { return info.getPhone(); }
string getCls() const { return info.getCls(); }
int getMathScore() const { return math; }
int getChineseScore() const { return chinese; }
int getEnglishScore() const { return english; }
double getAverageScore() const { return (math + chinese + english) / 3.0; }
private:
BasicInfo info;
int math;
int chinese;
int english;
};
// 教师类
class Teacher {
public:
Teacher(string name, string gender, string phone, string cls,
string major, int teachingYears) :
info(name, gender, phone, cls), major(major), teachingYears(teachingYears) {}
string getName() const { return info.getName(); }
string getGender() const { return info.getGender(); }
string getPhone() const { return info.getPhone(); }
string getCls() const { return info.getCls(); }
string getMajor() const { return major; }
int getTeachingYears() const { return teachingYears; }
private:
BasicInfo info;
string major;
int teachingYears;
};
int main() {
// 创建学生对象并调用成员函数
Student stu("小明", "男", "1234567890", "三年一班", 80, 90, 85);
cout << "姓名:" << stu.getName() << endl;
cout << "性别:" << stu.getGender() << endl;
cout << "手机号:" << stu.getPhone() << endl;
cout << "班级:" << stu.getCls() << endl;
cout << "数学成绩:" << stu.getMathScore() << endl;
cout << "语文成绩:" << stu.getChineseScore() << endl;
cout << "英语成绩:" << stu.getEnglishScore() << endl;
cout << "平均成绩:" << stu.getAverageScore() << endl;
// 创建教师对象并调用成员函数
Teacher teacher("张老师", "女", "0987654321", "三年一班", "数学", 5);
cout << "姓名:" << teacher.getName() << endl;
cout << "性别:" << teacher.getGender() << endl;
cout << "手机号:" << teacher.getPhone() << endl;
cout << "班级:" << teacher.getCls() << endl;
cout << "专业:" << teacher.getMajor() << endl;
cout << "教龄:" << teacher.getTeachingYears() << endl;
return 0;
}
```
在这个程序中,我们定义了三个类:`BasicInfo`、`Student`和`Teacher`。其中,`BasicInfo`类是一个基类,包含了姓名、性别、手机号和班级等基本信息;`Student`类和`Teacher`类都是通过组合技术来继承`BasicInfo`类,分别添加了学生和教师的特有成员变量和成员函数。
在`main`函数中,我们创建了一个学生对象和一个教师对象,并调用了它们的成员函数来输出它们的信息。
阅读全文
相关推荐
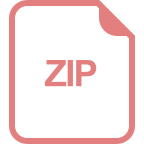
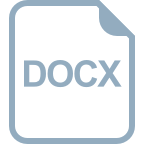
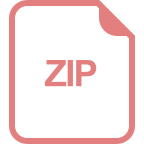















