用c++1.编写一个函数Print,能够打印多个学生的各门成绩,在主函数中定义一个可以存放有5个学生数据的数组。每个学生的数据包括num(学号),name(姓名),score[3](3门课的成绩)。在主函数输入这些数据,用Print函数输出这些数据。(结构体类型编写) 2.在上一题的基础上,编写一个函数SortStud对5个学生按每个学生的3门成绩总分(由高到低)进行排序,并用new申请存放5个学生数据的存储空间。
时间: 2024-04-06 07:33:16 浏览: 8
1. 代码如下:
```c++
#include <iostream>
using namespace std;
struct Student {
int num;
string name;
int score[3];
};
void Print(Student students[], int n) {
for (int i = 0; i < n; i++) {
cout << students[i].num << " " << students[i].name << " ";
for (int j = 0; j < 3; j++) {
cout << students[i].score[j] << " ";
}
cout << endl;
}
}
int main() {
const int n = 5;
Student students[n];
for (int i = 0; i < n; i++) {
cin >> students[i].num >> students[i].name;
for (int j = 0; j < 3; j++) {
cin >> students[i].score[j];
}
}
Print(students, n);
return 0;
}
```
2. 代码如下:
```c++
#include <iostream>
#include <algorithm>
using namespace std;
struct Student {
int num;
string name;
int score[3];
int total;
};
bool cmp(const Student& a, const Student& b) {
return a.total > b.total;
}
void SortStud(Student students[], int n) {
for (int i = 0; i < n; i++) {
students[i].total = 0;
for (int j = 0; j < 3; j++) {
students[i].total += students[i].score[j];
}
}
sort(students, students + n, cmp);
}
int main() {
const int n = 5;
Student* students = new Student[n];
for (int i = 0; i < n; i++) {
cin >> students[i].num >> students[i].name;
for (int j = 0; j < 3; j++) {
cin >> students[i].score[j];
}
}
SortStud(students, n);
for (int i = 0; i < n; i++) {
cout << students[i].num << " " << students[i].name << " " << students[i].total << endl;
}
delete[] students;
return 0;
}
```
这个程序定义了一个包含学生信息的结构体Student,其中包括学号、姓名、三门课的成绩和总成绩。首先定义了一个cmp函数,用于在SortStud函数中对学生按总成绩进行排序。SortStud函数中先计算每个学生的总成绩,然后调用sort函数按总成绩进行排序。在main函数中,首先动态申请了一个大小为5的Student数组,然后输入学生信息并调用SortStud函数进行排序,最后输出排序后的学生信息。注意在程序结束时需要用delete[]释放动态申请的内存空间。
相关推荐
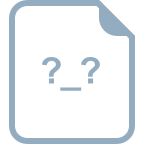
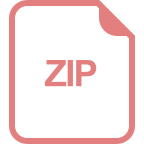
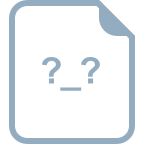









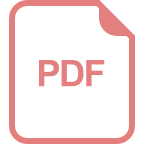
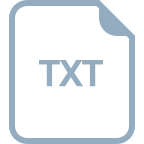
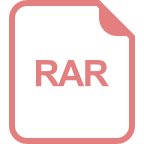
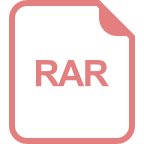