模拟实现动态分区分配中首次适应算法、循环首次适应算法、最佳适应算法和最坏适应算法。
时间: 2023-12-14 13:32:47 浏览: 63
以下是模拟实现动态分区分配中四种算法的Python代码:
1. 首次适应算法
```python
def first_fit(memory_blocks, process_size):
for i in range(len(memory_blocks)):
if memory_blocks[i] >= process_size:
memory_blocks[i] -= process_size
return True
return False
```
2. 循环首次适应算法
```python
def next_fit(memory_blocks, process_size, last_index):
for i in range(last_index, len(memory_blocks)):
if memory_blocks[i] >= process_size:
memory_blocks[i] -= process_size
return i
for i in range(last_index):
if memory_blocks[i] >= process_size:
memory_blocks[i] -= process_size
return i
return -1
```
3. 最佳适应算法
```python
def best_fit(memory_blocks, process_size):
best_index = -1
for i in range(len(memory_blocks)):
if memory_blocks[i] >= process_size:
if best_index == -1 or memory_blocks[i] < memory_blocks[best_index]:
best_index = i
if best_index != -1:
memory_blocks[best_index] -= process_size
return True
return False
```
4. 最坏适应算法
```python
def worst_fit(memory_blocks, process_size):
worst_index = -1
for i in range(len(memory_blocks)):
if memory_blocks[i] >= process_size:
if worst_index == -1 or memory_blocks[i] > memory_blocks[worst_index]:
worst_index = i
if worst_index != -1:
memory_blocks[worst_index] -= process_size
return True
return False
```
相关推荐
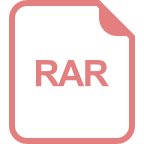
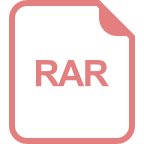








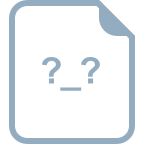
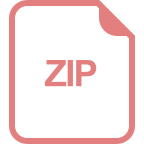
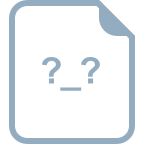
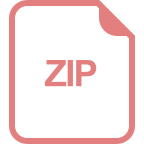
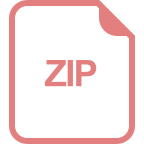