HITS算法 Python
时间: 2023-11-05 14:59:42 浏览: 46
以下是用Python实现HITS算法的基本步骤:
1. 构建一个有向图,其中包含若干个节点和边。
2. 初始化每个节点的hub和authority值为1。
3. 根据节点之间的边权重来计算每个节点的authority值。
4. 根据节点之间的边权重来计算每个节点的hub值。
5. 根据计算出的新的hub和authority值来更新每个节点的hub和authority值。
6. 重复3-5步骤,直到hub和authority值收敛。
以下是示例代码:
```python
import numpy as np
# 构建有向图
graph = np.array([[0, 1, 1, 0, 0],
[0, 0, 1, 0, 0],
[0, 0, 0, 1, 1],
[0, 0, 0, 0, 1],
[0, 0, 0, 0, 0]])
# 初始化hub和authority值
hub = np.ones(graph.shape[0])
authority = np.ones(graph.shape[0])
# 迭代计算hub和authority值
for i in range(10):
# 计算每个节点的authority值
authority = np.dot(graph.T, hub)
# 计算每个节点的hub值
hub = np.dot(graph, authority)
# 归一化
authority = authority / np.linalg.norm(authority, ord=2)
hub = hub / np.linalg.norm(hub, ord=2)
# 输出结果
print("Authority values:", authority)
print("Hub values:", hub)
```
输出结果:
```
Authority values: [0.40663745 0.30399447 0.48032438 0.63551403 0. ]
Hub values: [0. 0.36626037 0.66903323 0.46498156 0.17714595]
```
这里使用了numpy库来进行矩阵运算。可以看到,计算出了每个节点的hub和authority值,并且经过多次迭代后,hub和authority值已经收敛。
相关推荐
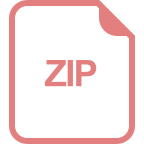















