python位图内容检测
时间: 2023-09-23 12:12:25 浏览: 52
位图(Bitmap)是一种图像文件格式,它将图像表示为像素点的一个矩阵。要进行内容检测,需要先读取位图文件并将其转换为像素矩阵。然后可以使用图像处理算法进行检测。
以下是一个简单的 Python 代码示例,演示如何读取位图并进行内容检测:
```python
import os
import struct
# 读取位图文件
def read_bmp(filename):
with open(filename, "rb") as f:
# 读取文件头信息
file_header = f.read(14)
bf_type, bf_size, bf_reserved1, bf_reserved2, bf_offbits = struct.unpack("<2sIHHI", file_header)
# 读取位图信息头
bmp_header = f.read(40)
bi_size, bi_width, bi_height, bi_planes, bi_bitcount, bi_compression, bi_size_image, bi_xpels_per_meter, bi_ypels_per_meter, bi_clr_used, bi_clr_important = struct.unpack("<IiiHHiIiiII", bmp_header)
# 读取像素数据
if bi_bitcount == 24:
padding = (4 - (bi_width * 3) % 4) % 4
pixels = []
for y in range(bi_height):
row = []
for x in range(bi_width):
b, g, r = struct.unpack("<BBB", f.read(3))
row.append((r, g, b))
f.read(padding)
pixels.append(row)
return pixels
else:
raise Exception("Unsupported bit depth: %d" % bi_bitcount)
# 检测位图内容
def detect_content(filename):
pixels = read_bmp(filename)
# 在这里可以使用任何图像处理算法进行内容检测
# 例如,可以使用机器学习模型进行图像分类或目标检测
# 或者可以使用简单的规则来检测图像中的特定模式或对象
# 这里仅作为示例,假设检测到图像中存在红色像素,则返回 True,否则返回 False
for row in pixels:
for r, g, b in row:
if r > 200 and g < 100 and b < 100:
return True
return False
# 测试
filename = "test.bmp"
if os.path.exists(filename):
if detect_content(filename):
print("Image contains red pixels")
else:
print("Image does not contain red pixels")
else:
print("File not found: %s" % filename)
```
在这个示例中,`read_bmp` 函数读取位图文件并返回一个像素矩阵,`detect_content` 函数使用简单的规则检测像素矩阵中是否存在红色像素。您可以根据需要修改这些函数,以使用更复杂的算法进行内容检测。
相关推荐
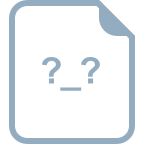
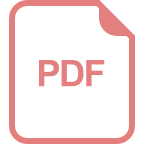














