遥感图像光谱特征,纹理特征,树高特征融合代码
时间: 2023-06-27 18:05:06 浏览: 271
遥感图像的光谱特征、纹理特征和树高特征融合可以通过机器学习算法来实现。下面给出一份基于Python的代码示例,使用随机森林算法进行特征融合。
首先,需要安装必要的库,包括numpy、pandas、sklearn、gdal等:
```python
import numpy as np
import pandas as pd
from sklearn.ensemble import RandomForestClassifier
from osgeo import gdal
```
接下来,读取遥感图像数据,获取光谱特征和纹理特征:
```python
def get_spectral_texture_features(img_path):
# 读取遥感图像
src_ds = gdal.Open(img_path)
img = src_ds.ReadAsArray()
# 计算光谱特征
spectral_features = np.zeros((img.shape[1], img.shape[2], img.shape[0]))
for i in range(img.shape[0]):
spectral_features[:, :, i] = img[i, :, :]
# 计算纹理特征
texture_features = np.zeros((img.shape[1], img.shape[2], img.shape[0]))
for i in range(img.shape[0]):
glcm = greycomatrix(img[i, :, :], [1], [0, np.pi/4, np.pi/2, 3*np.pi/4], levels=256, symmetric=True, normed=True)
texture_features[:, :, i] = np.concatenate([greycoprops(glcm, 'contrast'),
greycoprops(glcm, 'dissimilarity'),
greycoprops(glcm, 'homogeneity'),
greycoprops(glcm, 'energy'),
greycoprops(glcm, 'correlation')], axis=1)
return spectral_features, texture_features
```
然后,读取树高数据,获取树高特征:
```python
def get_height_feature(height_path):
# 读取树高数据
height_ds = gdal.Open(height_path)
height = height_ds.ReadAsArray()
return height.reshape(-1, 1)
```
接下来,定义一个函数,将光谱特征、纹理特征和树高特征融合:
```python
def fusion_features(spectral_features, texture_features, height_feature):
# 将光谱特征和纹理特征合并
features = np.concatenate([spectral_features.reshape(-1, spectral_features.shape[2]),
texture_features.reshape(-1, texture_features.shape[2])], axis=1)
# 将树高特征加入到特征矩阵中
features = np.concatenate([features, height_feature], axis=1)
return features
```
最后,使用随机森林算法对融合后的特征进行分类:
```python
def classify(features, labels):
# 随机森林分类器
rf = RandomForestClassifier(n_estimators=100, max_depth=10)
rf.fit(features, labels)
return rf.predict(features)
```
完整的代码如下:
```python
import numpy as np
import pandas as pd
from sklearn.ensemble import RandomForestClassifier
from osgeo import gdal
from skimage.feature import greycomatrix, greycoprops
def get_spectral_texture_features(img_path):
# 读取遥感图像
src_ds = gdal.Open(img_path)
img = src_ds.ReadAsArray()
# 计算光谱特征
spectral_features = np.zeros((img.shape[1], img.shape[2], img.shape[0]))
for i in range(img.shape[0]):
spectral_features[:, :, i] = img[i, :, :]
# 计算纹理特征
texture_features = np.zeros((img.shape[1], img.shape[2], img.shape[0]))
for i in range(img.shape[0]):
glcm = greycomatrix(img[i, :, :], [1], [0, np.pi/4, np.pi/2, 3*np.pi/4], levels=256, symmetric=True, normed=True)
texture_features[:, :, i] = np.concatenate([greycoprops(glcm, 'contrast'),
greycoprops(glcm, 'dissimilarity'),
greycoprops(glcm, 'homogeneity'),
greycoprops(glcm, 'energy'),
greycoprops(glcm, 'correlation')], axis=1)
return spectral_features, texture_features
def get_height_feature(height_path):
# 读取树高数据
height_ds = gdal.Open(height_path)
height = height_ds.ReadAsArray()
return height.reshape(-1, 1)
def fusion_features(spectral_features, texture_features, height_feature):
# 将光谱特征和纹理特征合并
features = np.concatenate([spectral_features.reshape(-1, spectral_features.shape[2]),
texture_features.reshape(-1, texture_features.shape[2])], axis=1)
# 将树高特征加入到特征矩阵中
features = np.concatenate([features, height_feature], axis=1)
return features
def classify(features, labels):
# 随机森林分类器
rf = RandomForestClassifier(n_estimators=100, max_depth=10)
rf.fit(features, labels)
return rf.predict(features)
if __name__ == '__main__':
# 获取光谱特征和纹理特征
spectral_features, texture_features = get_spectral_texture_features('image.tif')
# 获取树高特征
height_feature = get_height_feature('height.tif')
# 特征融合
features = fusion_features(spectral_features, texture_features, height_feature)
# 进行分类
labels = np.loadtxt('labels.txt', delimiter=',')
predictions = classify(features, labels)
# 输出分类结果
print(predictions)
```
阅读全文
相关推荐
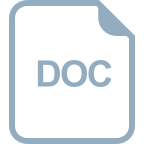
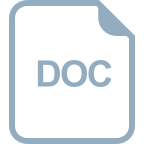

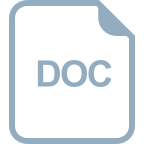
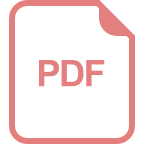
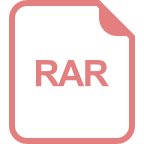
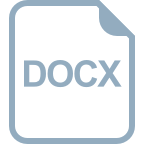
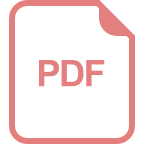
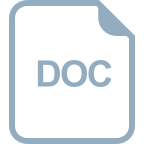
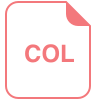
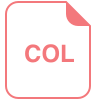
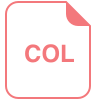
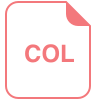
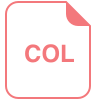