java springboot的分页查询
时间: 2023-10-12 20:55:48 浏览: 100
对于Java Spring Boot的分页查询,你可以按照以下步骤进行操作:
1. 在你的项目中添加Spring Data JPA依赖。可以在pom.xml文件中添加以下代码:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
```
2. 创建一个实体类,并使用`@Entity`和`@Table`注解标记。例如:
```java
@Entity
@Table(name = "your_table_name")
public class YourEntity {
// ...
}
```
3. 创建一个继承自`JpaRepository`的接口。例如:
```java
public interface YourRepository extends JpaRepository<YourEntity, Long> {
// ...
}
```
4. 在你的控制器(Controller)中注入`YourRepository`,并使用`Pageable`参数来实现分页查询。例如:
```java
@RestController
public class YourController {
private final YourRepository yourRepository;
public YourController(YourRepository yourRepository) {
this.yourRepository = yourRepository;
}
@GetMapping("/your-endpoint")
public Page<YourEntity> getEntities(Pageable pageable) {
return yourRepository.findAll(pageable);
}
}
```
5. 使用GET方法请求`/your-endpoint`,并在请求参数中指定页码和每页显示的数量,例如:
```
/your-endpoint?page=0&size=10
```
这样就可以实现基本的分页查询了。你可以根据需要在实体类和仓库接口中定义更多的查询方法,并结合分页查询来实现更复杂的功能。
阅读全文
相关推荐
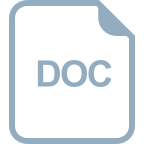
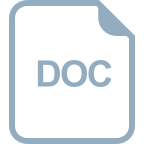
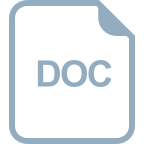

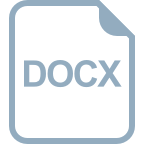
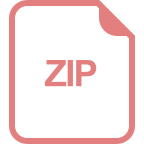











