Springboot 分页
时间: 2023-06-22 20:27:16 浏览: 103
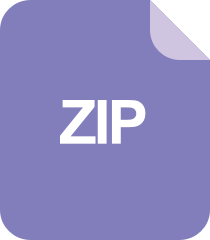
springBoot分页
在SpringBoot中使用分页功能可以借助MyBatis-Plus、Spring Data JPA等框架来实现。以下是使用MyBatis-Plus实现分页的示例:
1. 引入MyBatis-Plus依赖:
```xml
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>${mybatis-plus.version}</version>
</dependency>
```
2. 创建实体类和对应的Mapper接口:
```java
public class User {
private Long id;
private String name;
private Integer age;
// getter和setter省略
}
public interface UserMapper extends BaseMapper<User> {}
```
3. 在Mapper接口中定义方法:
```java
public interface UserMapper extends BaseMapper<User> {
IPage<User> selectUserPage(Page<User> page, @Param("name") String name);
}
```
4. 在对应的XML文件中实现方法:
```xml
<select id="selectUserPage" resultType="com.example.demo.entity.User">
select
id, name, age
from
user
<where>
<if test="name != null">
and name like concat('%', #{name}, '%')
</if>
</where>
</select>
```
5. 在Service层中调用Mapper接口方法:
```java
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserMapper userMapper;
@Override
public IPage<User> selectUserPage(Page<User> page, String name) {
return userMapper.selectUserPage(page, name);
}
}
```
6. 在Controller层中调用Service层方法:
```java
@RestController
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/users")
public IPage<User> selectUserPage(Page<User> page, String name) {
return userService.selectUserPage(page, name);
}
}
```
这样就完成了使用MyBatis-Plus实现SpringBoot分页的示例。其中,`Page<User>`是MyBatis-Plus提供的分页查询参数封装类,`IPage<User>`是MyBatis-Plus提供的分页查询结果封装类。在Controller层中,通过接收`Page<User>`参数来传递分页查询的相关参数,同时也可以传递其他的查询参数,比如上述示例中的`name`参数。
阅读全文
相关推荐
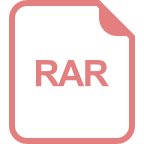














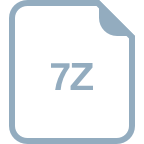