springboot分页功能实现
时间: 2023-10-13 15:18:13 浏览: 87
在Spring Boot中,可以通过使用Spring Data JPA来实现分页功能。Spring Data JPA是Spring框架的一个子项目,它提供了一种简化访问关系型数据库的方法。下面是一个简单的例子:
1. 添加依赖
在pom.xml文件中添加以下依赖:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
```
2. 创建实体类
创建一个实体类,并使用`@Entity`和`@Table`注解指定表名。在实体类中,可以使用`@Id`注解指定主键,以及其他注解来定义表的字段。
```
@Entity
@Table(name = "user")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String email;
// 省略getter和setter方法
}
```
3. 创建Repository
创建一个Repository接口,继承`JpaRepository`类,并使用`@Repository`注解标记。在Repository接口中,可以定义查询方法,以及排序和分页方法。
```
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
Page<User> findAll(Pageable pageable);
}
```
4. 实现分页功能
在Controller中,可以注入`UserRepository`接口,并调用`findAll(Pageable pageable)`方法来实现分页功能。下面是一个简单的例子:
```
@RestController
public class UserController {
@Autowired
private UserRepository userRepository;
@GetMapping("/users")
public Page<User> getUsers(@RequestParam(defaultValue = "0") int page,
@RequestParam(defaultValue = "10") int size) {
Pageable pageable = PageRequest.of(page, size);
return userRepository.findAll(pageable);
}
}
```
在上面的例子中,`@RequestParam`注解用于从请求参数中获取分页参数,`PageRequest.of`方法用于创建一个`Pageable`对象,`userRepository.findAll(pageable)`方法用于查询分页数据。
以上就是Spring Boot实现分页功能的基本步骤。
阅读全文
相关推荐
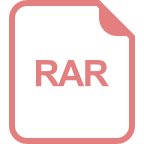
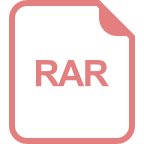
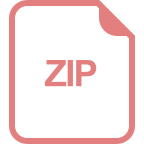














