springboot分页工具
时间: 2023-07-09 15:26:03 浏览: 43
Spring Boot 中可以使用 Spring Data JPA 提供的分页工具来实现分页功能,具体步骤如下:
1. 在 pom.xml 中添加 Spring Data JPA 和数据库驱动的依赖。
2. 在 Spring Boot 主类上添加 @EnableJpaRepositories 注解,启用 Spring Data JPA。
3. 创建一个继承自 JpaRepository 的接口,并在其中定义一个查询方法。
4. 在该查询方法的参数列表中添加 Pageable 类型的参数,用于接收分页参数。
5. 调用该查询方法时,传入一个 Pageable 对象,即可获取到分页后的数据。
具体代码实现可以参考以下示例:
```java
// 引入 Spring Data JPA 和数据库驱动的依赖
dependencies {
implementation 'org.springframework.boot:spring-boot-starter-data-jpa'
implementation 'mysql:mysql-connector-java'
}
// 启用 Spring Data JPA
@EnableJpaRepositories(basePackages = "com.example.demo.repository")
@SpringBootApplication
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
// 定义一个继承自 JpaRepository 的接口
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
// 定义一个查询方法,并添加 Pageable 类型的参数
Page<User> findAll(Pageable pageable);
}
// 调用查询方法,传入 Pageable 对象获取分页数据
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
public Page<User> findAll(int pageNum, int pageSize) {
Pageable pageable = PageRequest.of(pageNum, pageSize);
return userRepository.findAll(pageable);
}
}
```
相关推荐
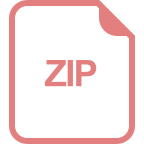
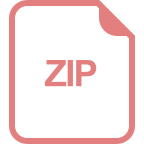














