springboot 带sum的分页
时间: 2023-09-15 16:05:54 浏览: 47
### 回答1:
Spring Boot中使用MyBatis-Plus实现带有SUM的分页查询可以按照以下步骤进行操作:
1. 添加MyBatis-Plus和PageHelper的依赖
在pom.xml文件中添加以下依赖:
```
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.4.0</version>
</dependency>
<dependency>
<groupId>com.github.pagehelper</groupId>
<artifactId>pagehelper-spring-boot-starter</artifactId>
<version>1.3.0</version>
</dependency>
```
2. 定义查询方法
在Mapper接口中定义查询方法,例如:
```java
@Select("SELECT sum(amount) as total_amount FROM orders")
List<AmountDTO> selectTotalAmount(Page<?> page);
```
3. 编写Controller
在Controller中调用查询方法并返回结果,例如:
```java
@GetMapping("/total-amount")
public PageResult<AmountDTO> getTotalAmount(@RequestParam(defaultValue = "1") int pageNum,
@RequestParam(defaultValue = "10") int pageSize) {
Page<?> page = PageHelper.startPage(pageNum, pageSize);
List<AmountDTO> list = orderMapper.selectTotalAmount(page);
return new PageResult<>(list, page.getTotal());
}
```
4. 定义返回结果
定义一个通用的分页返回结果类,例如:
```java
public class PageResult<T> {
private List<T> list;
private long total;
public PageResult(List<T> list, long total) {
this.list = list;
this.total = total;
}
// 省略getter和setter方法
}
```
这样就可以实现带有SUM的分页查询了。
### 回答2:
在Spring Boot中,可以使用Spring Data JPA来实现带sum的分页查询。
首先,需要创建一个JpaRepository接口的子接口,并继承PagingAndSortingRepository接口。在该接口中定义一个带有@Query注解的方法,可以在该注解中编写SQL查询语句,使用SUM函数计算总和,并进行分页。例如:
```java
@Repository
public interface UserRepository extends PagingAndSortingRepository<User, Long> {
@Query(value = "SELECT SUM(u.age) FROM User u WHERE u.salary > :minSalary")
Long sumAgeBySalary(@Param("minSalary") Double minSalary, Pageable pageable);
}
```
然后,在Service层调用该方法进行分页查询。例如:
```java
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
public Page<User> getUsersWithSumAgeBySalary(Double minSalary, int page, int size) {
Pageable pageable = PageRequest.of(page, size);
Long sumAge = userRepository.sumAgeBySalary(minSalary, pageable);
Page<User> users = userRepository.findAll(pageable);
users.forEach(user -> user.setSumAge(sumAge));
return users;
}
}
```
在上面的例子中,通过调用`userRepository.sumAgeBySalary(minSalary, pageable)`来获取总年龄和总工资满足条件的用户列表。然后,将总年龄设置到每个用户对象中。最后,返回分页的用户列表。
通过以上方法,就可以实现Spring Boot带有SUM的分页查询。
### 回答3:
Spring Boot是一个基于Spring框架的开发工具,它提供了开箱即用的功能,使得开发者可以更快、更方便地构建基于Java语言的应用程序。而带有sum的分页,通常是指在进行分页查询时,返回结果中同时包含总数量的功能。
在Spring Boot中,我们可以使用Spring Data JPA来实现带有sum的分页。首先,我们需要定义一个Repository接口,继承自JpaRepository,并添加自定义的查询方法。在查询方法上可以使用@Query注解,通过SQL或JPQL语句来实现自定义查询逻辑。例如:
```java
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
// 自定义查询方法,同时返回总数量
@Query(value = "select u from User u where u.age > ?1",
countQuery = "select count(u) from User u where u.age > ?1")
Page<User> findUsersByAgeGreaterThan(int age, Pageable pageable);
}
```
在上述代码中,我们定义了一个查询年龄大于指定值的用户的自定义查询方法。使用@Query注解,我们可以在value参数中指定查询语句,通过countQuery参数指定查询总数量的语句。Pageable参数用于实现分页,通过调用findUsersByAgeGreaterThan方法并传入Pageable对象,即可获取带有总数量的分页结果。
使用上述自定义的Repository接口,我们可以在Service层或Controller层进行调用,获取带有sum的分页结果。例如:
```java
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
public Page<User> getUsersByAgeGreaterThan(int age, int pageNum, int pageSize) {
Pageable pageable = PageRequest.of(pageNum, pageSize);
return userRepository.findUsersByAgeGreaterThan(age, pageable);
}
}
@RestController
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/users")
public Page<User> getUsers(@RequestParam int age,
@RequestParam int pageNum,
@RequestParam int pageSize) {
return userService.getUsersByAgeGreaterThan(age, pageNum, pageSize);
}
}
```
上述代码中,UserService类中的getUsersByAgeGreaterThan方法调用了UserRepository中自定义的查询方法,获取带有sum的分页结果。而UserController类中的getUsers方法使用了@GetMapping注解,接收前端传入的查询参数并调用UserService的方法,返回带有sum的分页结果。
通过上述的代码示例,我们可以在Spring Boot中实现带有sum的分页功能。这样,我们可以更方便地进行分页查询,并获取查询结果的总数量。
相关推荐
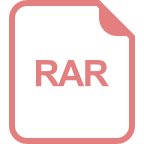
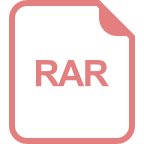
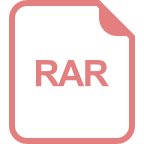














