c++写代码 请根据输入的逆序数据采用头插法创建一个单链表,然后删除相同的数据,仅保留一个。
时间: 2024-02-03 08:11:16 浏览: 52
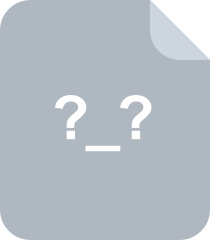
C++单链表的创建插入、删除

下面是头插法创建单链表并删除相同数据的示例代码:
```c++
#include <iostream>
using namespace std;
// 定义链表节点结构体
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(NULL) {}
};
// 头插法创建单链表
ListNode* createList(int n) {
ListNode* head = new ListNode(0);
for (int i = 0; i < n; i++) {
int val;
cin >> val;
ListNode* node = new ListNode(val);
node->next = head->next;
head->next = node;
}
return head->next;
}
// 删除相同数据
ListNode* deleteDuplicates(ListNode* head) {
if (!head) return head;
ListNode* cur = head;
while (cur->next) {
if (cur->val == cur->next->val) {
ListNode* tmp = cur->next;
cur->next = cur->next->next;
delete tmp;
} else {
cur = cur->next;
}
}
return head;
}
// 输出单链表
void printList(ListNode* head) {
while (head) {
cout << head->val << " ";
head = head->next;
}
cout << endl;
}
int main() {
int n;
cin >> n;
ListNode* head = createList(n);
head = deleteDuplicates(head);
printList(head);
return 0;
}
```
在上面的代码中,我们先通过头插法创建单链表,然后再对单链表进行删除相同数据的操作。最后输出删除相同数据后的单链表。
阅读全文
相关推荐







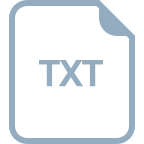
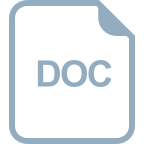
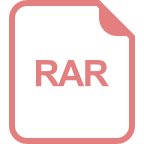
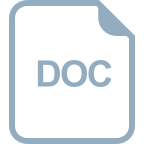
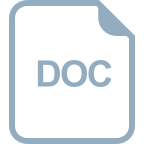
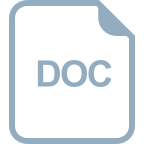
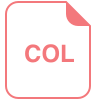
