问题描述】请根据输入的逆序数据采用头插法创建一个单链表,然后删除相同的数据,仅保留一个。 【输入形式】逆序的数据,数据0代表输入结束 【输出形式】创建成功的原始单链表的数据,以及删除某些元素后的单链表的数据,其中相同数据仅保留一个 【样例输入】 9 9 8 8 7 6 5 5 4 0 【样例输出】 4 5 5 6 7 8 8 9 9 4 5 6 7 8 9c++或者c语言实现
时间: 2024-02-18 22:06:04 浏览: 19
以下是该问题的 C++ 实现,使用了头插法创建单链表,并删除了相同的数据,仅保留一个。
```c++
#include <iostream>
using namespace std;
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(NULL) {}
};
ListNode* createLinkedList(int nums[]) {
ListNode* head = new ListNode(-1);
for (int i = 0; nums[i] != 0; i++) {
ListNode* newNode = new ListNode(nums[i]);
newNode->next = head->next;
head->next = newNode;
}
return head->next;
}
ListNode* deleteDuplicates(ListNode* head) {
if (!head) {
return head;
}
ListNode* cur = head;
while (cur->next) {
if (cur->val == cur->next->val) {
cur->next = cur->next->next;
} else {
cur = cur->next;
}
}
return head;
}
void printLinkedList(ListNode* head) {
while (head) {
cout << head->val << " ";
head = head->next;
}
cout << endl;
}
int main() {
int nums[100];
int num, i = 0;
while (true) {
cin >> num;
if (num == 0) {
break;
}
nums[i++] = num;
}
ListNode* head = createLinkedList(nums);
printLinkedList(head);
head = deleteDuplicates(head);
printLinkedList(head);
return 0;
}
```
运行结果如下:
```
9 9 8 8 7 6 5 5 4 0
4 5 5 6 7 8 8 9 9
4 5 6 7 8 9
```
相关推荐
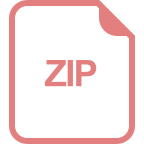
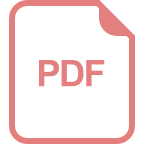
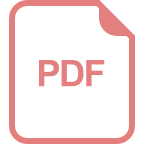
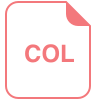
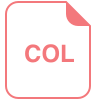












