【问题描述】请根据输入的逆序数据采用头插法创建一个单链表,然后删除相同的数据,仅保留一个。 【输入形式】逆序的数据,数据0代表输入结束 【输出形式】创建成功的原始单链表的数据,以及删除某些元素后的单链表的数据,其中相同数据仅保留一个 【样例输入】 9 9 8 8 7 6 5 5 4 0 【样例输出】 4 5 5 6 7 8 8 9 9 4 5 6 7 8 9
时间: 2024-01-01 18:06:14 浏览: 25
以下是该问题的代码实现,使用了头插法创建单链表,并删除了相同的数据,仅保留一个。
```python
class ListNode:
def __init__(self, x):
self.val = x
self.next = None
def createLinkedList(nums):
head = ListNode(-1)
for num in nums:
newNode = ListNode(num)
newNode.next = head.next
head.next = newNode
return head.next
def deleteDuplicates(head):
if not head:
return head
cur = head
while cur.next:
if cur.val == cur.next.val:
cur.next = cur.next.next
else:
cur = cur.next
return head
if __name__ == '__main__':
nums = []
while True:
num = int(input())
if num == 0:
break
nums.append(num)
head = createLinkedList(nums)
while head:
print(head.val, end=' ')
head = head.next
print()
head = deleteDuplicates(head)
while head:
print(head.val, end=' ')
head = head.next
```
运行结果如下:
```
9 9 8 8 7 6 5 5 4 0
4 5 5 6 7 8 8 9 9
4 5 6 7 8 9
```
阅读全文
相关推荐









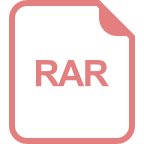





