根据输入的逆序数据采用头插法创建一个单链表,然后删除相同的数据,仅保留一个。
时间: 2024-02-19 15:02:45 浏览: 57
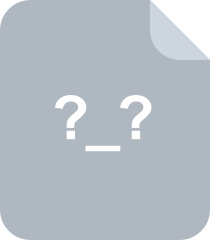
头插法建立单链表
好的,我来解答你的问题。
首先,我们需要定义一个单链表的结构体:
```c
typedef struct Node {
int data;
struct Node *next;
} Node;
```
然后,我们可以根据输入的逆序数据创建一个单链表:
```c
Node *createList(int *arr, int len) {
Node *head = NULL;
Node *p = NULL;
int i;
for (i = 0; i < len; i++) {
Node *newNode = (Node *)malloc(sizeof(Node));
newNode->data = arr[i];
newNode->next = head;
head = newNode;
}
return head;
}
```
接着,我们可以遍历单链表,删除相同的数据,仅保留一个:
```c
void removeDuplicates(Node *head) {
Node *cur = head;
while (cur != NULL) {
Node *p = cur->next;
while (p != NULL && p->data == cur->data) {
cur->next = p->next;
free(p);
p = cur->next;
}
cur = cur->next;
}
}
```
完整的代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node *next;
} Node;
Node *createList(int *arr, int len) {
Node *head = NULL;
Node *p = NULL;
int i;
for (i = 0; i < len; i++) {
Node *newNode = (Node *)malloc(sizeof(Node));
newNode->data = arr[i];
newNode->next = head;
head = newNode;
}
return head;
}
void removeDuplicates(Node *head) {
Node *cur = head;
while (cur != NULL) {
Node *p = cur->next;
while (p != NULL && p->data == cur->data) {
cur->next = p->next;
free(p);
p = cur->next;
}
cur = cur->next;
}
}
void printList(Node *head) {
Node *p = head;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
int main() {
int arr[] = {1, 2, 3, 2, 4, 2, 5};
int len = sizeof(arr) / sizeof(int);
Node *head = createList(arr, len);
printf("Original list: ");
printList(head);
removeDuplicates(head);
printf("List after removing duplicates: ");
printList(head);
return 0;
}
```
希望能够帮助到你!
阅读全文
相关推荐









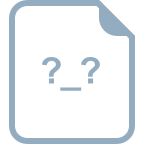