使用R语言实现对MNIST数据集识别,并在真实的手写数字上测试
时间: 2023-06-26 13:03:42 浏览: 106
好的,我可以为您提供一些关于如何使用R语言实现对MNIST数据集的识别的指导。
首先,您需要下载MNIST数据集。可以从以下网站下载:
http://yann.lecun.com/exdb/mnist/
接下来,您需要用R语言编写代码来处理和训练数据集。以下是一个基本的代码框架示例:
```
library(dplyr)
library(tidyr)
library(ggplot2)
library(reshape2)
library(caret)
library(randomForest)
# Load the MNIST dataset
train = read.table("path/to/train.csv", header=TRUE, sep=",")
test = read.table("path/to/test.csv", header=TRUE, sep=",")
# Convert the data to data frames
train_df = data.frame(train)
test_df = data.frame(test)
# Split the training data into training and validation sets
set.seed(123)
trainIndex <- createDataPartition(train_df$label, p=0.8, list=FALSE)
train_data <- train_df[trainIndex, ]
valid_data <- train_df[-trainIndex, ]
# Train a random forest model on the training data
rf_model <- randomForest(label ~ ., data=train_data, ntree=500)
# Make predictions on the validation data
valid_pred <- predict(rf_model, newdata=valid_data)
# Calculate accuracy on the validation data
accuracy <- mean(valid_pred == valid_data$label)
print(paste("Validation accuracy:", accuracy))
# Make predictions on the test data
test_pred <- predict(rf_model, newdata=test_df)
# Write the predictions to a CSV file for submission
write.csv(test_pred, file="path/to/submission.csv", row.names=FALSE)
```
在这个示例中,我们首先使用`read.table()`函数从CSV文件中读取MNIST数据集。然后,我们将数据转换为数据帧,并使用`createDataPartition()`函数将训练数据分成训练和验证集。接下来,我们训练了一个随机森林模型,并在验证数据上进行了预测和评估。最后,我们使用该模型对测试数据进行了预测,并将预测结果写入CSV文件以进行提交。
请注意,这只是一个基本的示例,您可能需要对代码进行调整和优化以达到更好的结果。此外,您需要为此代码提供正确的文件路径和文件名。
最后,您可以使用真实的手写数字测试您的模型。您可以将数字扫描或拍照,然后使用类似于MNIST数据集的方式对其进行处理和预测。
阅读全文
相关推荐
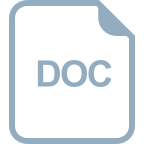
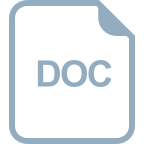
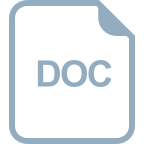
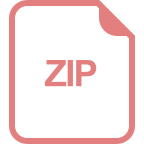
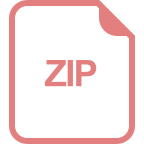
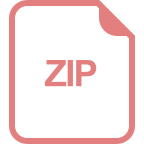
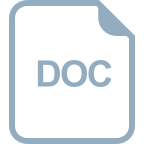




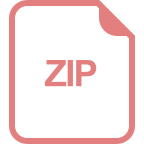
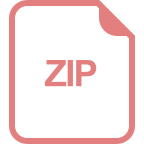
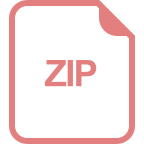
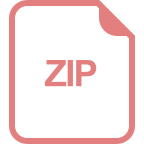
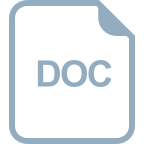


