用C++编写求二叉树的叶节点数、总节点数和深度的代码
时间: 2024-05-01 21:17:25 浏览: 42
以下是求二叉树叶节点数、总节点数和深度的 C 代码:
```
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
int data;
struct node* left;
struct node* right;
} Node;
int leaf_count(Node* root) {
if (root == NULL) {
return 0;
}
if (root->left == NULL && root->right == NULL) {
return 1;
} else {
return leaf_count(root->left) + leaf_count(root->right);
}
}
int node_count(Node* root) {
if (root == NULL) {
return 0;
} else {
return 1 + node_count(root->left) + node_count(root->right);
}
}
int depth(Node* root) {
if (root == NULL) {
return 0;
} else {
int left_depth = depth(root->left);
int right_depth = depth(root->right);
if (left_depth > right_depth) {
return left_depth + 1;
} else {
return right_depth + 1;
}
}
}
Node* create_node(int data) {
Node* node = (Node*) malloc(sizeof(Node));
node->data = data;
node->left = NULL;
node->right = NULL;
return node;
}
int main() {
Node* root = create_node(1);
root->left = create_node(2);
root->right = create_node(3);
root->left->left = create_node(4);
root->left->right = create_node(5);
root->right->left = create_node(6);
root->right->right = create_node(7);
printf("Leaf node count of binary tree: %d\n", leaf_count(root));
printf("Total node count of binary tree: %d\n", node_count(root));
printf("Depth of binary tree: %d\n", depth(root));
return 0;
}
```
阅读全文
相关推荐
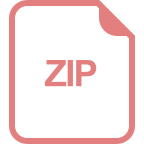


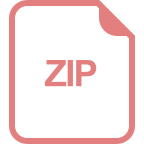
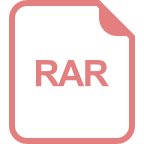
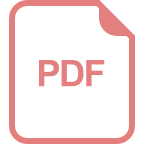
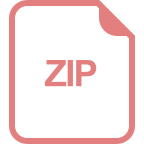
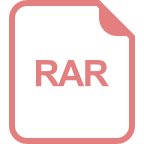
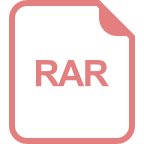
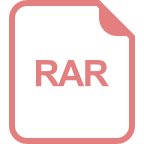
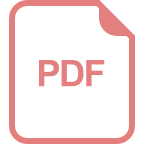
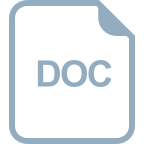
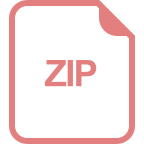
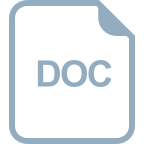
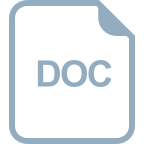
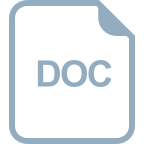