python爬取雅虎图片
时间: 2023-09-14 10:11:35 浏览: 55
下面是一份Python代码,可以爬取Yahoo图片并保存到本地:
```python
import requests
from bs4 import BeautifulSoup
import urllib.request
url = "https://www.yahoo.com/news/photos"
response = requests.get(url)
soup = BeautifulSoup(response.text, "html.parser")
image_tags = soup.find_all("img")
for image_tag in image_tags:
image_url = image_tag.get("src")
if "https" in image_url:
image_name = image_url.split("/")[-1]
urllib.request.urlretrieve(image_url, image_name)
print(f"Image saved: {image_name}")
```
这段代码使用了Requests和BeautifulSoup库来获取网页内容和解析HTML。然后,它使用urllib库将图片保存到本地。
相关问题
python爬取金融数据
要爬取金融数据,你可以使用 Python 中的 Requests 库和 BeautifulSoup 库来获取和解析网页数据。以下是一个简单的示例代码,可以爬取雅虎财经的股票数据:
```python
import requests
from bs4 import BeautifulSoup
# 获取股票代码为 AAPL 的股票数据
url = 'https://finance.yahoo.com/quote/AAPL'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# 获取当前股价
current_price = soup.find('span', {'data-reactid': '50'}).text
print('当前股价:', current_price)
# 获取股票历史价格
history_prices = []
history = soup.find_all('td', {'class': 'Py(10px) Pstart(10px)'})
for data in history:
history_prices.append(data.find('span').text)
print('历史股价:', history_prices)
```
在这个示例中,我们使用 Requests 库来请求雅虎财经的 AAPL 股票网页数据,并使用 BeautifulSoup 库来解析网页数据。然后,我们可以使用 find() 和 find_all() 方法来查找特定的网页元素,并从中提取所需的数据。
python金融数据爬取代码
你好,以下是 Python 爬取金融数据的示例代码:
```python
import requests
import pandas as pd
url = 'https://finance.yahoo.com/quote/AAPL/history?p=AAPL'
response = requests.get(url)
table = pd.read_html(response.text)[0]
table = table.iloc[::-1]
table = table.reset_index(drop=True)
print(table)
```
这段代码可以爬取雅虎财经中苹果公司(AAPL)的历史股价数据。
相关推荐
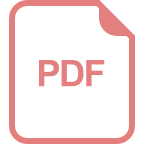
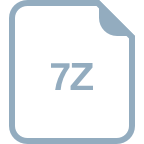
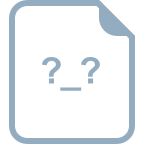






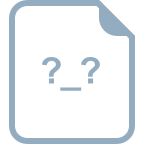
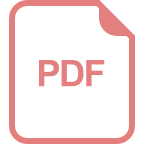
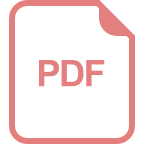
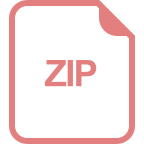
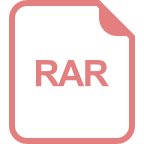