#include <iostream> using namespace std; template<class numtype> class Compare {public: Compare(numtype a,numtype b); numtype max(); numtype min(); private: numtype x,y; }; template <class numtype> Compare<numtype>::Compare(numtype a,numtype b) {x=a;y=b;} template <class numtype> numtype Compare<numtype>::max() {return (x>y)?x:y;} template <class numtype> numtype Compare<numtype>::min() {return (x<y)?x:y;} int main() {Compare<int> cmp1(3,7); cout<<cmp1.max()<<" is the Maximum of two integer numbers."<<endl; cout<<cmp1.min()<<" is the Minimum of two integer numbers."<<endl<<endl; Compare<float> cmp2(45.78,93.6); cout<<cmp2.max()<<" is the Maximum of two float numbers."<<endl; cout<<cmp2.min()<<" is the Minimum of two float numbers."<<endl<<endl; Compare<char> cmp3('a','A'); cout<<cmp3.max()<<" is the Maximum of two characters."<<endl; cout<<cmp3.min()<<" is the Minimum of two characters."<<endl; return 0; }
时间: 2023-10-30 19:00:17 浏览: 44
这段代码实现了一个模板类 Compare,用于比较两个数的大小并输出最大值和最小值。模板类 Compare 中包含一个 numtype 类型的模板参数,用于表示要比较的数的类型。类中定义了构造函数,用于初始化对象的成员变量 x 和 y。类中还定义了两个成员函数 max 和 min,分别用于求出两个数中的最大值和最小值。在主函数中,分别创建了三个 Compare 对象 cmp1、cmp2 和 cmp3,分别用于比较两个整数、两个浮点数以及两个字符的大小,并输出它们的最大值和最小值。最终输出的结果为:
7 is the Maximum of two integer numbers.
3 is the Minimum of two integer numbers.
93.6 is the Maximum of two float numbers.
45.78 is the Minimum of two float numbers.
a is the Maximum of two characters.
A is the Minimum of two characters.
相关问题
#include<iostream> #include<cstdio> using namespace std;是什么意思
#include<iostream> #include<cstdio> using namespace std; 是C++中的预处理指令,用于引入头文件和命名空间。
1. #include<iostream> 是引入iostream头文件,其中包含了输入输出流的定义,例如cout和cin等。
2. #include<cstdio> 是引入cstdio头文件,其中包含了C语言标准输入输出函数的定义,例如printf和scanf等。
3. using namespace std; 是使用std命名空间,std是C++标准库的命名空间,其中包含了很多常用的函数和类。
这些预处理指令的作用是为了在程序中能够使用输入输出流和标准库函数,使得程序更加方便和简洁。
#include<iostream>using namespace std;
这是一个C++的代码片段,它包含了iostream头文件和使用了命名空间std。iostream头文件提供了输入输出流的功能,而using namespace std;语句则是为了方便使用std命名空间中的函数和对象,避免每次都需要写std::。
以下是一个简单的示例代码,展示了如何使用iostream头文件和命名空间std来输出一句话:
```cpp
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
```
这段代码会输出"Hello, World!",endl是一个特殊的控制符,用于在输出流中插入一个换行符。
相关推荐
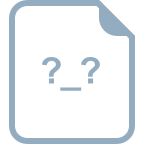













