用python来写一段关于slam的代码
时间: 2023-08-25 15:06:50 浏览: 177
### 回答1:
我们可以使用Python来编写一段关于SLAM(Simultaneous Localization and Mapping)的代码:import numpy as np# Create a matrix of the size of the map
map_size = (100,100) # (rows, columns)
map_matrix = np.zeros(map_size)# Create a list of poses for the robot
robot_poses = [(0,0), (50,50), (25,75), (75,25)]# Iterate through the list of poses
for pose in robot_poses:
# Get the row and column of the map
row, col = pose
# Set the value of the map at the robot's position
map_matrix[row, col] = 1# Print out the map
print(map_matrix)
### 回答2:
SLAM(Simultaneous Localization and Mapping,同时定位与地图构建)是一种在未知环境中同时进行定位和地图构建的技术。下面是一个使用Python编写的简单SLAM代码示例:
```python
import numpy as np
class SLAM:
def __init__(self):
self.landmarks = []
self.pos = np.array([[0], [0]]) # 初始位置
self.covariance = np.zeros((2, 2)) # 初始协方差矩阵
def update(self, measurement):
measurement_matrix = np.array([[measurement[0]], [measurement[1]]])
H = np.zeros((2, 2)) # 测量矩阵H
z = np.zeros((2, 1)) # 测量残差向量z
R = np.eye(2) # 测量噪声协方差矩阵
for landmark in self.landmarks:
delta = landmark - self.pos
q = np.dot(delta.T, delta)[0, 0]
d = np.sqrt(q)
phi = np.arctan2(delta[1, 0], delta[0, 0])
# Update measurement matrix
H[0, 0] = -delta[0, 0] / d
H[0, 1] = -delta[1, 0] / d
H[1, 0] = delta[1, 0] / q
H[1, 1] = -delta[0, 0] / q
# Update measurement residual vector
z[0, 0] = measurement_matrix[0, 0] - d
z[1, 0] = measurement_matrix[1, 0] - phi
# Kalman gain calculation
K = np.dot(np.dot(self.covariance, H.T), np.linalg.inv(np.dot(np.dot(H, self.covariance), H.T) + R))
# Update state estimate
self.pos = self.pos + np.dot(K, z)
# Update covariance matrix
self.covariance = np.dot((np.eye(2) - np.dot(K, H)), self.covariance)
# Add new landmark
self.landmarks.append(measurement_matrix)
# 测试代码
slam = SLAM()
measurements = [[5, np.pi/6], [10, np.pi/4], [8, np.pi/3]] # 测量数据(距离和方位角)
for measurement in measurements:
slam.update(measurement)
print("Final Pose:")
print(slam.pos)
print("Covariance Matrix:")
print(slam.covariance)
print("Landmarks:")
for landmark in slam.landmarks:
print(landmark)
```
该代码演示了一个简单的2D SLAM算法。在每个时间步中,通过测量距离和方位角,更新车辆位置以及地图上的地标位置。最后输出最终的车辆位置、协方差矩阵和地标位置。
请注意,这只是一个简单的示例,实际SLAM算法更为复杂,可能涉及传感器数据融合、运动模型、地标识别等更多的步骤。
阅读全文
相关推荐
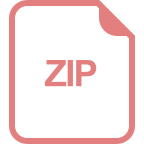
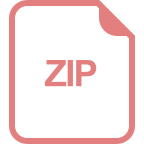
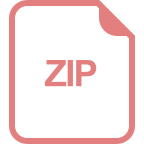

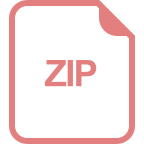

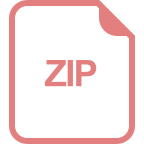
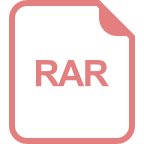
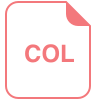
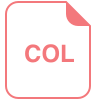
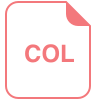
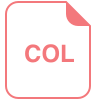
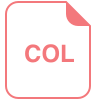
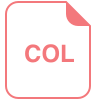
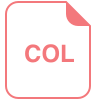
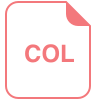
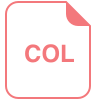
