如何解决c++中的n皇后问题
时间: 2024-04-30 10:17:57 浏览: 80
n皇后问题是一个经典的计算机科学问题,它的目标是在一个nxn的棋盘上放n个皇后,使得每个皇后都无法攻击到其他皇后。这个问题可以通过递归回溯算法来解决。
首先,我们定义一个函数solveNQueens,它会接受当前要放置的行数和当前已经保存的解决方案。对于每一行,我们遍历每一列,尝试将皇后放在该位置。如果该位置合法,我们就将皇后放在该位置,并递归调用solveNQueens函数,然后再回溯到该位置,移除皇后。如果我们无法找到合法的位置,则回溯到上一行。
以下是一个简单的C代码实现:
```
#include <stdio.h>
#include <stdbool.h>
/* 棋盘尺寸 */
#define N 8
/* 使用全局变量保存解决方案 */
int solution[N];
/* 检查当前位置是否合法 */
bool is_valid(int row, int col) {
for (int i = 0; i < row; i++) {
if (solution[i] == col || solution[i] - i == col - row || solution[i] + i == col + row) {
return false;
}
}
return true;
}
/* 递归回溯函数 */
void solveNQueens(int row) {
if (row >= N) {
/* 打印解决方案 */
for (int i = 0; i < N; i++) {
for (int j = 0; j < N; j++) {
if (solution[i] == j) {
printf("Q ");
} else {
printf(". ");
}
}
printf("\n");
}
printf("\n");
return;
}
for (int col = 0; col < N; col++) {
if (is_valid(row, col)) {
solution[row] = col;
solveNQueens(row + 1);
solution[row] = -1;
}
}
}
/* 主函数 */
int main() {
/* 初始化解决方案 */
for (int i = 0; i < N; i++) {
solution[i] = -1;
}
/* 开始搜索 */
solveNQueens(0);
return 0;
}
```
此代码会打印出所有8皇后问题的解决方案,每个方案都表示为一个NxN的棋盘。其中,皇后用大写字母Q表示,空格用小数点表示。
阅读全文
相关推荐

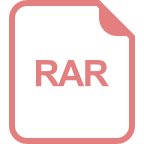
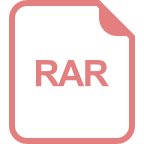
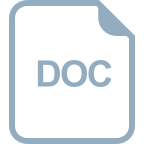
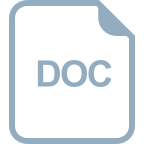
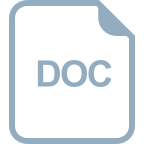
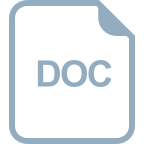
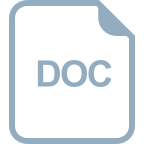
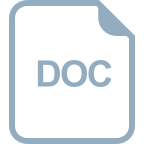
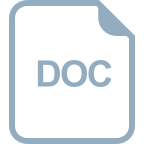
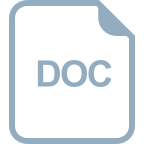
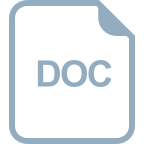
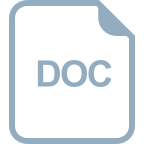
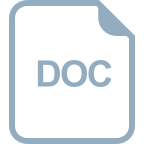

