c++读取带逗号的文件
时间: 2024-10-14 21:17:04 浏览: 26
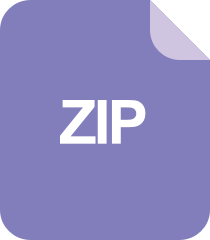
C++_C++_读取txt文档_txt内容读取_按空格分隔_按逗号分隔_

在C++中,读取包含逗号分隔值(CSV, Comma Separated Values)的文件通常涉及到文本文件操作和数据解析。你可以使用标准库中的`fstream`或`ifstream`类来读取文件,同时配合字符串处理来分割每一行的数据。以下是一个简单的示例:
```cpp
#include <fstream>
#include <sstream> // 使用istringstream处理字符串流
#include <vector> // 存储每行数据
std::vector<std::string> read_csv(const std::string& filename) {
std::ifstream file(filename);
if (!file.is_open()) {
throw std::runtime_error("Failed to open the file");
}
std::vector<std::string> data;
std::string line;
while (getline(file, line)) {
std::istringstream iss(line); // 创建输入流从行开始
std::string cell;
std::vector<std::string> row;
while (iss >> cell) { // 分割逗号直到遇到空格或文件结束
row.push_back(cell);
if (!iss.peek() || iss.peek() == ',') { // 如果遇到非数字且不是下一个字段,则认为这一行已结束
iss.ignore(); // 忽略剩余的逗号
break;
}
}
data.push_back(row); // 将行数据添加到结果向量中
}
return data;
}
// 示例使用:
int main() {
try {
const std::vector<std::vector<std::string>>& csv_data = read_csv("your_file.csv");
for (const auto& row : csv_data) {
for (const auto& cell : row) {
std::cout << cell << ", ";
}
std::cout << "\n";
}
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << '\n';
}
return 0;
}
```
阅读全文
相关推荐
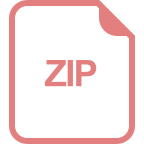
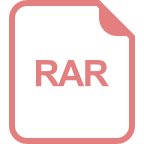

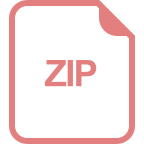
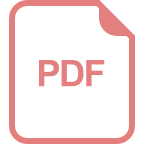
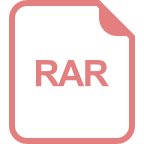











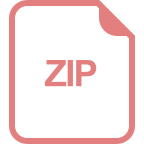