c++ 读取txt用逗号分隔的每一行数据
时间: 2024-05-16 08:16:10 浏览: 130
假设你的 txt 文件长这样:
```
1,2,3,4,5
6,7,8,9,10
11,12,13,14,15
```
你可以使用 C++ 的 `std::ifstream` 类和 `std::getline` 函数来读取每行数据,并使用 `std::istringstream` 类和 `std::getline` 函数来将每行数据用逗号分隔成多个字段。
以下是一个例子:
```cpp
#include <iostream>
#include <fstream>
#include <sstream>
#include <string>
#include <vector>
int main() {
// 打开文件
std::ifstream file("data.txt");
if (!file.is_open()) {
std::cerr << "Failed to open file!" << std::endl;
return -1;
}
// 读取每行数据
std::string line;
while (std::getline(file, line)) {
// 分隔每行数据
std::istringstream iss(line);
std::string field;
std::vector<std::string> fields;
while (std::getline(iss, field, ',')) {
fields.push_back(field);
}
// 打印每个字段
for (const auto& field : fields) {
std::cout << field << " ";
}
std::cout << std::endl;
}
// 关闭文件
file.close();
return 0;
}
```
输出结果:
```
1 2 3 4 5
6 7 8 9 10
11 12 13 14 15
```
注:代码中使用了 `std::vector` 类来存储每行数据的多个字段。如果你不想使用这个类,你可以使用数组或其他容器来存储这些字段。
阅读全文
相关推荐

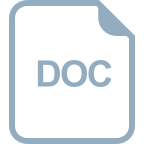






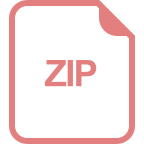






