c++读取txt点云数据
时间: 2023-08-23 12:08:19 浏览: 175
读取txt点云数据可以使用Python中的NumPy库和Pandas库。以下是一些示例代码:
使用NumPy:
```python
import numpy as np
# 读取点云数据
data = np.loadtxt('point_cloud.txt')
# 查看数据的形状
print(data.shape)
# 查看前5个数据
print(data[:5])
```
使用Pandas:
```python
import pandas as pd
# 读取点云数据
data = pd.read_csv('point_cloud.txt', header=None, delim_whitespace=True)
# 查看数据的形状
print(data.shape)
# 查看前5行数据
print(data.head())
```
注意,以上代码中的`point_cloud.txt`是你的点云数据文件名,需要根据实际情况进行更改。另外,如果你的点云数据文件包含列名,则可以将`header=None`改为`header=0`。如果你的数据文件是用空格分隔的而不是用逗号分隔的,则可以将`delim_whitespace=True`改为`sep=' '`。
相关问题
c++pcl读取txt点云数据
使用PCL库可以很方便地读取txt格式的点云数据。以下是一个简单的示例代码:
```cpp
#include <pcl/io/pcd_io.h>
#include <pcl/point_types.h>
int main (int argc, char** argv)
{
// 创建点云对象
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud (new pcl::PointCloud<pcl::PointXYZ>);
// 读取txt文件
if (pcl::io::loadPCDFile<pcl::PointXYZ> ("filename.txt", *cloud) == -1) //* 读入文件
{
PCL_ERROR ("Couldn't read file filename.txt \n");
return (-1);
}
// 输出点云信息
std::cout << "Loaded "
<< cloud->width * cloud->height
<< " data points from filename.txt with the following fields: "
<< std::endl;
// 遍历点云并输出xyz坐标
for (size_t i = 0; i < cloud->points.size (); ++i)
std::cout << " " << cloud->points[i].x
<< " " << cloud->points[i].y
<< " " << cloud->points[i].z << std::endl;
return (0);
}
```
在读取txt文件时,可以使用`loadPCDFile`函数,参数为文件名和点云对象指针。如果读取文件失败,函数返回-1。成功读取文件后,可以通过点云对象的`points`成员访问点云中的所有点,并输出其xyz坐标。
c++读写txt点云 逗号分隔 具体举例并进行详细注释
在C++中,处理文本文件(如.txt格式的CSV点云数据,其中点由逗号分隔)通常涉及到文件输入流(ifstream)用于读取,和文件输出流(ofstream)用于写入。这里是一个简单的例子,展示如何读取和写入CSV格式的数据:
```cpp
#include <fstream>
#include <string>
#include <vector>
// 定义一个结构体表示一个三维点
struct Point {
double x;
double y;
double z;
};
// 读取CSV文件函数
std::vector<Point> read_txt_point_cloud(const std::string& filename) {
std::ifstream file(filename);
if (!file.is_open()) {
throw std::runtime_error("Failed to open file: " + filename);
}
std::vector<Point> points;
std::string line;
while (std::getline(file, line)) { // 逐行读取
if (line.empty() || line[0] == '#') continue; // 跳过空行和注释
// 使用stringstream将每一行分割成x, y, z
std::stringstream ss(line);
std::string part;
Point p;
if (!(ss >> part >> p.x >> part >> p.y >> part >> p.z)) {
throw std::runtime_error("Invalid data format in file");
}
points.push_back(p); // 将点添加到点云中
}
file.close();
return points;
}
// 写入CSV文件函数
void write_txt_point_cloud(const std::vector<Point>& points, const std::string& filename) {
std::ofstream output_file(filename);
if (!output_file.is_open()) {
throw std::runtime_error("Failed to create file: " + filename);
}
for (const auto& point : points) {
output_file << point.x << "," << point.y << "," << point.z << "\n"; // 写入每个点,换行分隔
}
output_file.close();
}
int main() {
try {
std::vector<Point> cloud = read_txt_point_cloud("input.txt");
write_txt_point_cloud(cloud, "output.txt");
std::cout << "Data successfully processed!" << std::endl;
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
```
在这个例子中,`read_txt_point_cloud`函数负责从给定的CSV文件中读取点云数据,并存储在一个`Point`类型的向量中。而`write_txt_point_cloud`函数则将这个点云以相同的格式写入另一个文件。
阅读全文
相关推荐
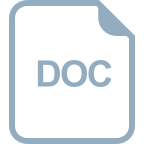
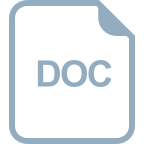
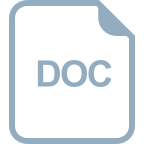


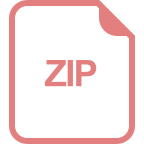
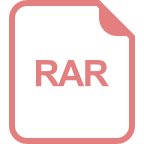








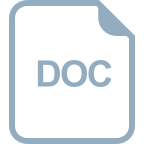