c语言实现使用两个 线程来模拟 生产者和消费者生产十个消费十个,并使用了 pthread 库提供的线程操作,需要包含 头文件<pthread.h>
时间: 2024-10-11 20:13:56 浏览: 34
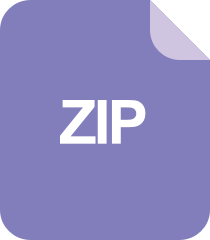
(Linux C)利用多进程或多线程模拟实现生产者/消费者问题
在C语言中,我们可以使用`pthread`库来创建并管理线程,模拟生产者和消费者模型。下面是一个简单的示例,展示了如何创建两个线程,一个作为生产者负责生成10个元素放入队列,另一个作为消费者负责从队列中取出并处理这10个元素:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <semaphore.h> // 使用信号量sem_t控制同步
#define QUEUE_SIZE 10
sem_t empty; // 当队列为空时,空信号量计数为1
sem_t full; // 当队列满时,满信号量计数为0
int queue[QUEUE_SIZE]; // 队列用于存储生产者的元素
int count = 0; // 记录队列中实际的元素数量
void* producer(void* arg) {
int i;
for (i = 0; i < 10; ++i) {
sem_wait(&full); // 等待队列不满
printf("Producer produced element %d\n", i);
queue[count++] = i; // 元素入队
sem_post(&empty); // 队列不满变为队列满
}
return NULL;
}
void* consumer(void* arg) {
int i;
for (i = 0; i < 10; ++i) {
sem_wait(&empty); // 等待队列不空
printf("Consumer consumed element %d from queue\n", queue[i]);
queue[i] = -1; // 消费者处理后清零位置
sem_post(&full); // 队列不空变为队列满
}
return NULL;
}
int main() {
pthread_t producer_thread, consumer_thread;
if (pthread_create(&producer_thread, NULL, producer, NULL) != 0) {
perror("Error creating producer thread");
exit(1);
}
if (pthread_create(&consumer_thread, NULL, consumer, NULL) != 0) {
perror("Error creating consumer thread");
exit(1);
}
pthread_join(producer_thread, NULL);
pthread_join(consumer_thread, NULL);
return 0;
}
阅读全文
相关推荐
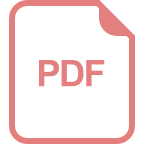
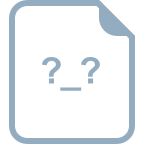





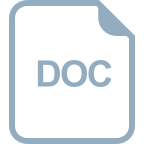









