写一个脚本,可以对某网站进行遍历,下载所有pdf; 添加异常处理、加入多线程;自定义 UserAgentMiddleware,并使用 ip 代理来健壮我们的爬虫程序;每读取一个网页,等待随机0~5秒后再执行下一个操作
时间: 2024-03-28 20:37:47 浏览: 122
好的,我来回答你的问题。
首先,需要安装 `requests`、`beautifulsoup4` 和 `fake_useragent` 库。
然后,可以使用以下代码来实现对某网站进行遍历,下载所有 pdf 文件:
```python
import os
import random
import requests
from bs4 import BeautifulSoup
from fake_useragent import UserAgent
from retrying import retry
from concurrent.futures import ThreadPoolExecutor
@retry(stop_max_attempt_number=3)
def get_response(url):
headers = {'User-Agent': UserAgent().random}
proxies = {'http': 'http://your_ip:your_port', 'https': 'https://your_ip:your_port'}
response = requests.get(url, headers=headers, proxies=proxies, timeout=10)
response.raise_for_status()
return response
def download_pdf(url, path):
response = get_response(url)
with open(path, 'wb') as f:
f.write(response.content)
def traverse_website(url):
response = get_response(url)
soup = BeautifulSoup(response.text, 'html.parser')
for link in soup.find_all('a'):
href = link.get('href')
if href.endswith('.pdf'):
pdf_url = url + href
pdf_name = href.split('/')[-1]
pdf_path = os.path.join('pdfs', pdf_name)
if os.path.exists(pdf_path):
print(f'{pdf_path} already exists!')
else:
print(f'Downloading {pdf_name}...')
download_pdf(pdf_url, pdf_path)
def main():
urls = ['http://example.com/page1', 'http://example.com/page2', 'http://example.com/page3']
with ThreadPoolExecutor(max_workers=5) as executor:
for url in urls:
executor.submit(traverse_website, url)
time.sleep(random.randint(0, 5))
if __name__ == '__main__':
main()
```
这段代码使用了 `requests` 库来发送 HTTP 请求,`beautifulsoup4` 库来解析 HTML,`fake_useragent` 库来生成随机的 User-Agent,`retrying` 库来添加异常处理和重试机制,`ThreadPoolExecutor` 来实现多线程下载,以及 `time` 库来实现随机等待。
其中,`get_response` 函数用来发送 HTTP 请求并返回响应,如果请求失败会进行重试;`download_pdf` 函数用来下载 pdf 文件;`traverse_website` 函数用来遍历网站并下载所有 pdf 文件;`main` 函数用来启动多线程下载,并在每次下载后随机等待0~5秒。
在使用时,需要将 `your_ip` 和 `your_port` 替换为你的代理 IP 和端口号,并将 `urls` 替换为你要遍历的网站页面链接列表。
阅读全文
相关推荐
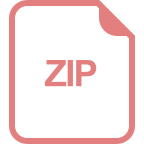
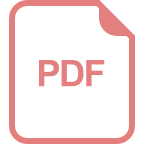
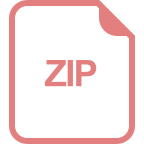
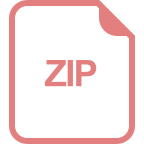
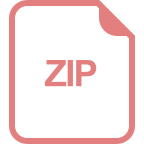
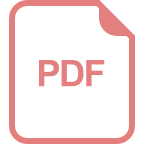
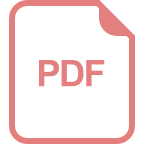
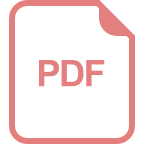
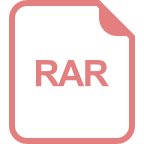
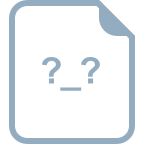
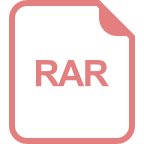
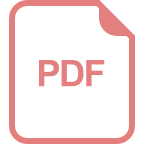
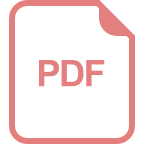
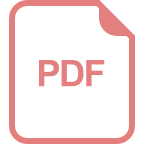
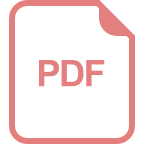
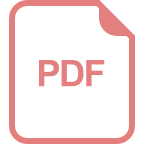