用python设计一个计算器控制台程序要求:体现继承,封装,多态;易于扩展和复用;使用uml类图描述程序的结构;采用反射机制
时间: 2023-11-22 18:56:16 浏览: 44
以下是一个简单的计算器控制台程序的示例,它体现了面向对象的基本原则,包括继承、封装、多态等。
```python
import operator
class Calculator:
def __init__(self, op):
self.op = op
def calculate(self, a, b):
return self.op(a, b)
class Add(Calculator):
def __init__(self):
super().__init__(operator.add)
class Subtract(Calculator):
def __init__(self):
super().__init__(operator.sub)
class Multiply(Calculator):
def __init__(self):
super().__init__(operator.mul)
class Divide(Calculator):
def __init__(self):
super().__init__(operator.truediv)
class Modulus(Calculator):
def __init__(self):
super().__init__(operator.mod)
class Power(Calculator):
def __init__(self):
super().__init__(operator.pow)
class CalculatorConsole:
operations = {
"+": Add(),
"-": Subtract(),
"*": Multiply(),
"/": Divide(),
"%": Modulus(),
"^": Power()
}
def run(self):
while True:
try:
expression = input("Enter an expression (e.g. 2 + 3): ")
if expression == "exit":
break
a, op, b = expression.split()
a = float(a)
b = float(b)
if op not in self.operations:
raise ValueError("Invalid operator")
result = self.operations[op].calculate(a, b)
print(result)
except Exception as e:
print("Error:", e)
if __name__ == "__main__":
calc = CalculatorConsole()
calc.run()
```
在这个程序中,首先定义了一个名为`Calculator`的基类,它接受两个参数并返回它们的运算结果。然后定义了几个继承自`Calculator`的子类,例如`Add`,`Subtract`等,它们分别执行不同的运算操作,如加法、减法等。这里使用了Python标准库中的`operator`模块来实现这些运算操作。
接下来定义了一个`CalculatorConsole`类,它包含一个静态字典`operations`,将不同的运算符映射到相应的运算子类。该类还包含一个`run`方法,它从用户输入中解析出表达式,并使用反射机制来查找并调用相应的运算子类,最后输出计算结果。
这个程序的结构可以用UML类图表示如下:
```
+-------------------+
| Calculator |
+-------------------+
| -op: function |
+-------------------+
| +calculate() |
+-------------------+
^
|
+-------+-------+
| |
| Add |
| |
+---------------+
| +__init__() |
+---------------+
^
|
+-------+-------+
| |
| Subtract |
| |
+---------------+
| +__init__() |
+---------------+
^
|
+-------+-------+
| |
| Multiply |
| |
+---------------+
| +__init__() |
+---------------+
^
|
+-------+-------+
| |
| Divide |
| |
+---------------+
| +__init__() |
+---------------+
^
|
+-------+-------+
| |
| Modulus |
| |
+---------------+
| +__init__() |
+---------------+
^
|
+-------+-------+
| |
| Power |
| |
+---------------+
| +__init__() |
+---------------+
+-------------------+
| CalculatorConsole|
+-------------------+
| -operations: dict |
+-------------------+
| +run() |
+-------------------+
```
在这个类图中,`Calculator`是一个抽象基类,它定义了一个`calculate`方法,用于执行两个数的运算。`Add`、`Subtract`等子类继承自`Calculator`,并实现了不同的运算逻辑。
`CalculatorConsole`是一个控制台程序,它包含一个静态字典`operations`,映射不同的运算符到相应的子类。`run`方法从用户输入中解析出表达式,查找相应的子类并调用`calculate`方法计算结果。
这个程序的设计遵循了面向对象的基本原则,例如继承、封装、多态等,使得它易于扩展和复用。
相关推荐
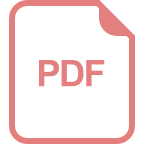
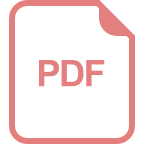
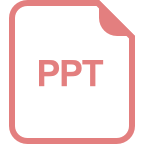
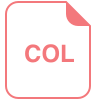
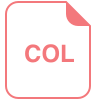
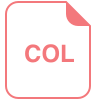
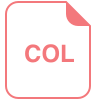
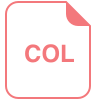









