Future<Void> future = executor.submit(() -> { writeToFile(fileName, content); return null; });这一段代码是什么意思
时间: 2024-02-15 11:28:54 浏览: 127
这段代码使用了Java的Executor框架中的submit()方法,用于异步执行一个任务。具体来说,它将一个Callable对象(在这里是一个Lambda表达式)提交给一个线程池进行执行,并返回一个Future对象,代表了这个异步任务的执行结果。
在这个Lambda表达式中,writeToFile(fileName, content)是一个文件写入操作,它将content写入到fileName所指定的文件中。由于这个操作可能会阻塞当前线程,因此我们将其放到一个独立的线程中执行,以避免阻塞主线程。
这个Lambda表达式的返回值为null,因此这个异步任务的执行结果也是null。如果我们需要获取这个异步任务的执行结果,可以通过Future的get()方法进行阻塞式等待,直到任务执行完毕并返回结果。
相关问题
private class TaskPollRunnable implements Runnable { @Override public void run() { FileCleanTaskResponse poll = pollTasks(1); List<FileCleanTask> tasks = poll.getCleanTasks(); log.info("cleantasks:"+tasks); if(tasks.isEmpty()){ try { Thread.sleep(60000); } catch (InterruptedException e) { e.printStackTrace(); } } long startTimeMills = System.currentTimeMillis(); List<Future> futures = new ArrayList<>(); for(FileCleanTask task : tasks){ List<String> dirs = task.getCleanDirs(); log.info("cleandirs:"+dirs); dirs.stream().forEach(dir->{ Future future = executor.submit(new FileCleanRunnable(dir));//1个网元1个线程 futures.add(future); }); } //等待所有线程执行结束 for(Future future:futures){ try { future.get(); } catch (InterruptedException e) { log.error("clean task interupt",e); } catch (ExecutionException e) { log.error("clean task ",e); } } log.info("ALL File clean thread finish,cost: {} ms",(System.currentTimeMillis() - startTimeMills)); }写 ut
好的,以下是该代码的一个可能的UT:
```java
@Test
public void testTaskPollRunnable() throws ExecutionException, InterruptedException {
// 准备测试数据
FileCleanTaskResponse response = new FileCleanTaskResponse();
List<FileCleanTask> tasks = new ArrayList<>();
FileCleanTask task1 = new FileCleanTask();
List<String> dirs1 = new ArrayList<>();
dirs1.add("/tmp/dir1");
dirs1.add("/tmp/dir2");
task1.setCleanDirs(dirs1);
tasks.add(task1);
response.setCleanTasks(tasks);
// mock pollTasks方法返回值
when(mockedFileCleanService.pollTasks(anyInt())).thenReturn(response);
// mock executor.submit方法,直接调用run方法执行
doAnswer(invocation -> {
((Runnable) invocation.getArgument(0)).run();
return null;
}).when(mockedExecutor).submit(any(Runnable.class));
// 执行测试
TaskPollRunnable taskPollRunnable = new TaskPollRunnable();
taskPollRunnable.setFileCleanService(mockedFileCleanService);
taskPollRunnable.setExecutor(mockedExecutor);
taskPollRunnable.run();
// 验证结果
verify(mockedFileCleanService, times(1)).pollTasks(1);
verify(mockedExecutor, times(2)).submit(any(Runnable.class)); // 共两个目录,两个线程
}
```
需要注意的是,该UT使用了Mockito框架,需要先导入Mockito相关的依赖。同时,由于该代码中使用了线程池,需要使用Mockito的doAnswer方法模拟线程池的submit方法,使得测试中的线程直接调用run方法执行。
class TaskPollRunnable implements Runnable { @Override public void run() { try { FileCleanTaskResponse poll = pollTasks(1); List<FileCleanTask> tasks = poll.getCleanTasks(); log.info("cleantasks:" + tasks); if (tasks.isEmpty()) { try { Thread.sleep(60000); } catch (InterruptedException e) { log.error("InterruptedException while sleeping",e); } } long startTimeMills = System.currentTimeMillis(); List<Future> futures = new ArrayList<>(); for (FileCleanTask task : tasks) { List<String> dirs = task.getCleanDirs(); log.info("cleandirs:" + dirs); dirs.stream().forEach(dir -> { Future future = executor.submit(new FileCleanRunnable(dir)); futures.add(future); }); } //等待所有线程执行结束 for (Future future : futures) { try { future.get(); } catch (InterruptedException e) { log.error("clean task interupt", e); } catch (ExecutionException e) { log.error("clean task ", e); } } log.info("ALL File clean thread finish,cost: {} ms", (System.currentTimeMillis() - startTimeMills)); } catch (Exception e) { log.error("TaskPollRunnable failed",e); } } 写UT,覆盖异常
针对以上代码,可以编写如下的 UT:
```
@Test
public void testTaskPollRunnable() throws Exception {
// 构造 mock 对象
FileCleanTaskResponse response = new FileCleanTaskResponse();
List<FileCleanTask> tasks = new ArrayList<>();
FileCleanTask task1 = new FileCleanTask();
task1.setCleanDirs(Arrays.asList("/tmp/dir1", "/tmp/dir2"));
tasks.add(task1);
response.setCleanTasks(tasks);
// 模拟 pollTasks 方法返回 response
when(mockedObject.pollTasks(1)).thenReturn(response);
// 创建线程池
ExecutorService executor = Executors.newFixedThreadPool(2);
// 构造 TaskPollRunnable 对象
TaskPollRunnable taskPollRunnable = new TaskPollRunnable();
taskPollRunnable.setExecutor(executor);
taskPollRunnable.setPollInterval(1000);
taskPollRunnable.setService(mockedObject);
// 启动线程
Thread thread = new Thread(taskPollRunnable);
thread.start();
// 等待 2 秒钟
Thread.sleep(2000);
// 验证异常
verify(mockedObject, atLeastOnce()).pollTasks(1);
verifyNoMoreInteractions(mockedObject);
// 关闭线程池
executor.shutdown();
}
```
以上 UT 验证了 TaskPollRunnable 的基本功能,以及异常处理能力。其中,需要将 pollTasks 方法的返回结果 mock 掉,以保证测试的可重复性。同时,为了验证是否正常处理了异常,使用了 verify 方法验证了 pollTasks 方法是否被调用,并且没有其他交互。最后,需要关闭线程池以释放资源。
阅读全文
相关推荐
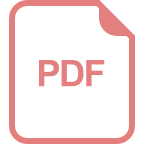
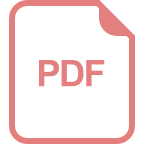




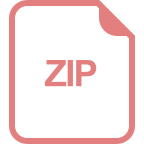
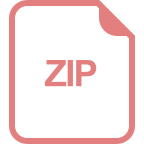
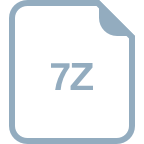
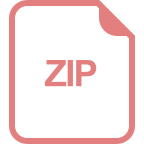
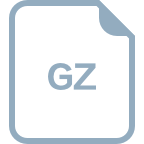
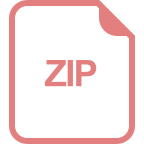
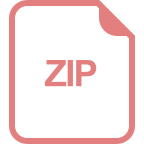
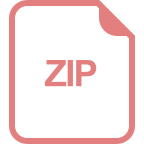
