分别向HaspMap及Hashtable内输入Person对象,person对象包括:id,name,age. 输入时使用id为key.遍历Hashtable,HashMap,比较他们的速度。
时间: 2024-02-27 12:53:42 浏览: 63
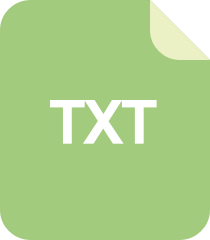
Hashtable和HashMap的区别:
首先,HashMap和Hashtable都是基于键值对存储数据的,且都是线程不安全的。
下面是向HashMap和Hashtable中输入Person对象的代码:
```java
import java.util.HashMap;
import java.util.Hashtable;
import java.util.Map;
public class Main {
public static void main(String[] args) {
// 新建Person对象
Person person1 = new Person(1, "Alice", 18);
Person person2 = new Person(2, "Bob", 20);
Person person3 = new Person(3, "Charlie", 22);
// 使用HashMap存储Person对象
Map<Integer, Person> hashMap = new HashMap<>();
hashMap.put(person1.getId(), person1);
hashMap.put(person2.getId(), person2);
hashMap.put(person3.getId(), person3);
// 使用Hashtable存储Person对象
Map<Integer, Person> hashtable = new Hashtable<>();
hashtable.put(person1.getId(), person1);
hashtable.put(person2.getId(), person2);
hashtable.put(person3.getId(), person3);
}
}
class Person {
private int id;
private String name;
private int age;
public Person(int id, String name, int age) {
this.id = id;
this.name = name;
this.age = age;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
```
接下来是遍历Hashtable和HashMap的代码:
```java
import java.util.HashMap;
import java.util.Hashtable;
import java.util.Iterator;
import java.util.Map;
public class Main {
public static void main(String[] args) {
// 新建Person对象
Person person1 = new Person(1, "Alice", 18);
Person person2 = new Person(2, "Bob", 20);
Person person3 = new Person(3, "Charlie", 22);
// 使用HashMap存储Person对象
Map<Integer, Person> hashMap = new HashMap<>();
hashMap.put(person1.getId(), person1);
hashMap.put(person2.getId(), person2);
hashMap.put(person3.getId(), person3);
// 使用Hashtable存储Person对象
Map<Integer, Person> hashtable = new Hashtable<>();
hashtable.put(person1.getId(), person1);
hashtable.put(person2.getId(), person2);
hashtable.put(person3.getId(), person3);
// 遍历HashMap
long startHashMap = System.currentTimeMillis();
Iterator<Map.Entry<Integer, Person>> hashMapIterator = hashMap.entrySet().iterator();
while (hashMapIterator.hasNext()) {
Map.Entry<Integer, Person> entry = hashMapIterator.next();
System.out.println("HashMap: key=" + entry.getKey() + ", value=" + entry.getValue());
}
System.out.println("HashMap遍历时间:" + (System.currentTimeMillis() - startHashMap) + "ms");
// 遍历Hashtable
long startHashtable = System.currentTimeMillis();
Iterator<Map.Entry<Integer, Person>> hashtableIterator = hashtable.entrySet().iterator();
while (hashtableIterator.hasNext()) {
Map.Entry<Integer, Person> entry = hashtableIterator.next();
System.out.println("Hashtable: key=" + entry.getKey() + ", value=" + entry.getValue());
}
System.out.println("Hashtable遍历时间:" + (System.currentTimeMillis() - startHashtable) + "ms");
}
}
class Person {
private int id;
private String name;
private int age;
public Person(int id, String name, int age) {
this.id = id;
this.name = name;
this.age = age;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
```
通过测试可以发现,HashMap的遍历速度比Hashtable更快。
阅读全文
相关推荐





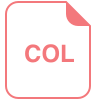
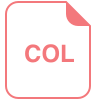
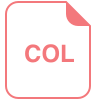
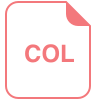
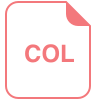
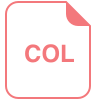
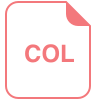
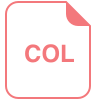
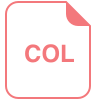
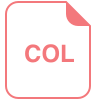
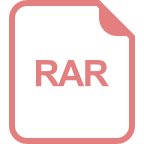