Java Map异常处理宝典:常见Map异常的理解与应对策略
发布时间: 2024-09-11 06:39:46 阅读量: 55 订阅数: 46 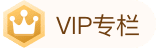
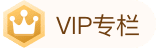
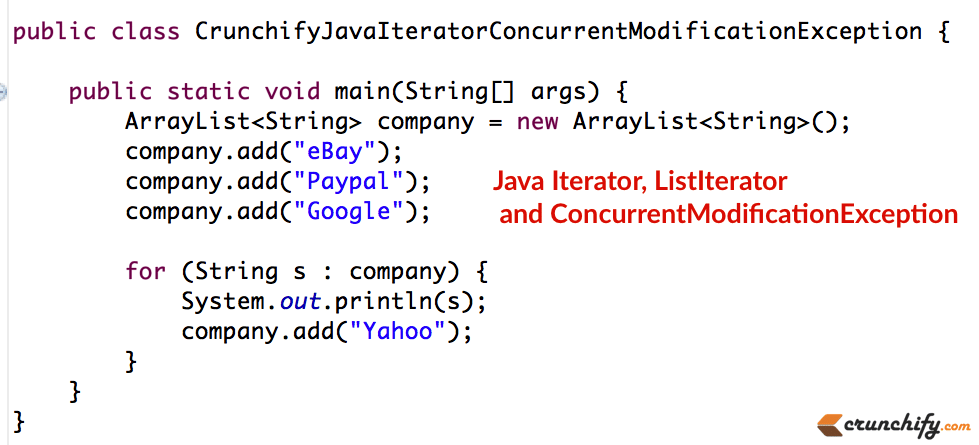
# 1. Java Map接口概述
Java Map接口是Java集合框架的一部分,它存储键值对,允许我们通过键快速检索数据。Map不同于List,它不保证顺序,而是侧重于数据项之间的关联。本章将对Map接口的基本概念进行介绍,探讨它的核心功能以及如何在Java程序中使用Map。
## 1.1 Map的基本概念
Map是一个存储键值对的数据结构,它的设计目标是通过唯一的键来快速检索对应的值。Map的实现类包括HashMap, TreeMap, Hashtable等,每个类都提供了特定的性能特性和使用场景。
## 1.2 Map的使用方式
使用Map很简单,通常包括以下几个步骤:
- 创建Map实例。
- 使用put方法添加键值对。
- 通过键来获取值。
- 遍历Map中的键值对。
例如:
```java
Map<String, String> map = new HashMap<>();
map.put("key1", "value1");
String value = map.get("key1");
for (Map.Entry<String, String> entry : map.entrySet()) {
System.out.println(entry.getKey() + " - " + entry.getValue());
}
```
## 1.3 Map的关键特性
Map接口提供了一套丰富的操作方法,例如:
- `size()`: 返回Map中键值对的数量。
- `isEmpty()`: 检查Map是否为空。
- `containsKey(Object key)`: 检查Map是否包含特定的键。
- `remove(Object key)`: 移除Map中的键值对。
理解Map接口的使用是进行复杂数据结构操作的基础,下一章节我们将深入探讨与Map操作相关的异常问题。
# 2. 深入理解Map异常
### 2.1 Map异常的类型与分类
Map接口在Java集合框架中扮演着重要的角色,它提供了一种存储键值对的方式。然而在实际应用中,开发者可能会遇到与Map操作相关的异常。Map异常可以分类为运行时异常和编译时异常,以帮助我们更好地理解和处理这些潜在问题。
#### 2.1.1 运行时异常
运行时异常通常指的是在Java程序运行时可能会遇到的异常,它们通常不是由程序员可以预料到的。在Map操作中,典型的运行时异常包括但不限于`NullPointerException`、`ClassCastException`等。
```java
Map<String, Object> myMap = new HashMap<>();
myMap.put(null, "value"); // 这里会抛出NullPointerException
```
在上面的代码示例中,如果调用者没有正确检查键或值是否为`null`,就可能会触发`NullPointerException`。运行时异常的特点是它们不被强制要求捕获或声明,因此它们在运行时才会被抛出。
#### 2.1.2 编译时异常
编译时异常,也被称为检查型异常,需要在编译阶段进行处理。这意味着要么捕获这些异常,要么声明它们将被抛出。例如,当我们尝试打开一个不存在的文件作为输入流时,会遇到`FileNotFoundException`。
```java
FileInputStream fis = new FileInputStream("non_existent_file.txt"); // 这里会抛出FileNotFoundException
```
编译时异常的处理要求在代码中显式地捕获或声明,这样做可以提前发现潜在的问题,有助于编写更健壮的代码。
### 2.2 Map异常的触发场景
Map接口的实现非常广泛,包括`HashMap`、`TreeMap`等。不同的实现方法在处理数据时可能会引发不同的异常场景。
#### 2.2.1 空指针异常与Map操作
在使用Map时,可能会忘记初始化或者在操作前没有检查元素是否为`null`,从而导致空指针异常。如下面的代码所示:
```java
Map<String, String> myMap = new HashMap<>();
String key = null;
String value = myMap.get(key); // 这里会抛出NullPointerException
```
这个例子中,如果没有对`key`是否为`null`进行检查就调用`get`方法,就会引发空指针异常。
#### 2.2.2 类型转换异常与Map转换
当尝试将一个对象从一种类型转换为另一种不兼容的类型时,可能会抛出`ClassCastException`。以下是一个简单的例子:
```java
Map<String, Object> map = new HashMap<>();
map.put("key", 123);
String value = (String) map.get("key"); // 这里会抛出ClassCastException
```
在这个例子中,`map.get("key")`返回的是一个`Integer`类型,但是尝试将其强制转换为`String`类型,这导致了类型转换异常。
### 2.3 Map异常的内部机制解析
深入理解Map异常的内部机制对于有效处理这些异常至关重要。异常处理的关键是异常的抛出与捕获机制以及异常链的使用。
#### 2.3.1 异常的抛出与捕获机制
当方法中发生了异常情况,且当前方法无法处理时,异常会被抛出。Java提供了`try-catch-finally`语句块来捕获和处理这些异常。开发者可以在`try`块中编写可能会抛出异常的代码,并在`catch`块中处理异常。`finally`块则用于执行必须执行的代码,无论是否发生异常。
```java
try {
// 可能会抛出异常的代码
} catch (ExceptionType1 e1) {
// 处理异常1
} catch (ExceptionType2 e2) {
// 处理异常2
} finally {
// 无论是否发生异常,都会执行的代码
}
```
异常的抛出与捕获机制确保了异常可以被适当地处理,防止程序因未处理的异常而意外终止。
#### 2.3.2 异常链与异常信息的重要性
异常链是指一个异常通过构造函数被传给另一个异常,从而创建了一个异常链。这有助于在抛出新的异常时保持原始异常的信息,使得问题的调试和追踪变得更加容易。异常对象的`getCause()`方法可以用来访问原始异常。
异常信息在处理异常时同样重要,它不仅帮助开发者了解发生了什么类型的错误,还能提供错误发生的具体环境和条件。因此,在记录和报告异常时,应当尽可能提供详细和精确的异常信息。
以上内容构成了本文第二章的核心内容,深入解析了Java Map异常的类型、触发场景以及内部机制。后续章节将继续深入探讨如何处理这些异常,以及如何通过工具和框架优化异常处理流程,从而提升Java应用的健壮性和性能。
# 3. Java Map异常处理最佳实践
## 3.1 异常处理原则
### 3.1.1 遵循异常处理的黄金法则
在Java编程中,异常处理是一个重要的方面,它能够帮助开发者管理程序运行时遇到的问题。异常处理的黄金法则之一是尽量捕获具体异常。这样做可以确保只有预期的错误被处理,而不是随意捕获所有异常,这可能导致隐藏了其他问题或者程序的逻辑错误。
```java
try {
// 操作可能会抛出多种异常的代码
} catch (SpecificException1 ex) {
// 处理特定类型的异常
} catch (SpecificException2 ex) {
// 处理另一种特定类型的异常
} catch (Exception ex) {
// 最后捕获通用异常,通常作为兜底
}
```
在上述代码中,`SpecificException1`和`SpecificException2`分别代表特定类型的异常,这样根据异常的类型采取相应的处理措施。`Exception`则是所有异常的基类,通常是最后的兜底处理,以确保所有未被前面捕捉的异常也能得到处理。
### 3.1.2 避免使用过时的异常处理方法
随着Java版本的更新,一些异常处理方法和实践被证明是低效或者不推荐使用的。一个典型的例子是使用`printStackTrace()`方法。这个方法虽然简单,但是它会将异常信息输出到标准错误流,并不会提供任何额外的错误处理或者日志记录功能。因此,更推荐使用日志框架来记录异常信息。
```java
try {
// 操作可能引发异常的代码
} catch (Exception ex) {
// 使用日志记录异常信息
log.error("发生异常,原因: {}", ex.getMessage());
}
```
这里,`log.error()`方法是记录错误信息的标准做法,它将异常信息记录到日志文件中,而不是仅仅打印到控制台。这种做法有助于系统管理员或者开发者追踪和分析问题。
## 3.2 常用的异常处理技术
### 3.2.1 try-catch-finally结构的应用
`try-catch-finally`是Java中处理异常的核心结构。`try`块包含了可能发生异常的代码。`catch`块跟随在`try`块之后,并且包含了处理异常的代码。`finally`块则是无论是否发生异常都会执行的代码块,通常用于清理资源。
```java
FileReader fileReader = null;
try {
fileReader = new FileReader("file.txt");
// 读取文件的操作可能抛出IOException
} catch (FileNotFoundException e) {
// 文件未找到异常的处理
} catch (IOException e) {
// 其他IO异常的处理
} finally {
// 关闭资源,无论是否发生异常
if (fileReader != null) {
try {
fileReader.close();
} catch (IOException ex) {
// 关闭失败的处理,通常是记录日志
log.error("关闭文件时发生异常", ex);
}
}
}
```
### 3.2.2 异常处理的性能影响与优化
异常处理是需要消耗一定资源的,特别是当异常频繁抛出和捕获时。虽然在某些情况下异常处理能提高代码的健壮性,但过度依赖异常处理会损害程序性能。
优化措施包括:
- 减少异
0
0
相关推荐
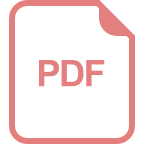
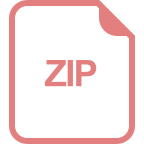
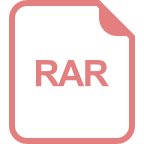





