Java Map遍历技巧揭秘:快速高效遍历Map的方法大公开
发布时间: 2024-09-11 06:11:24 阅读量: 67 订阅数: 37 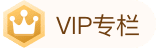
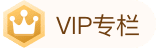
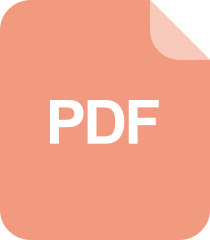
java遍历Map的几种方法分析

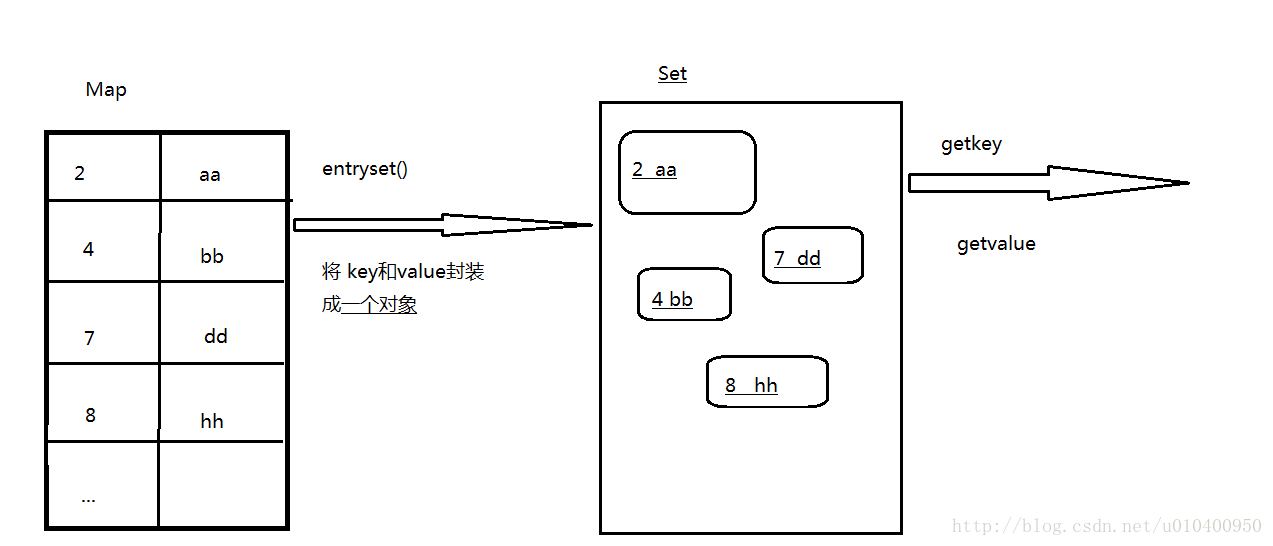
# 1. Java Map遍历的基本概念
在Java编程中,Map是一个存储键值对的集合,其中每个键都与一个值相关联。Map集合提供了多种遍历方式,以便开发人员可以遍历其内容,进行数据处理和操作。遍历Map意味着访问其所有的键值对,这在数据处理和查询中非常常见。本章将介绍Java Map遍历的基础知识,为理解和掌握后续章节的深入内容奠定基础。
# 2. Java Map遍历的理论基础
### 2.1 Map接口的结构与遍历机制
#### 2.1.1 Map接口的核心方法与实现
Java Map 是一个存储键值对(key-value pairs)的接口,提供了一系列用于管理这些键值对的方法。其中核心方法包括 `put(K key, V value)`, `get(Object key)`, `remove(Object key)` 以及 `containsKey(Object key)` 等。实现 Map 接口的常用类有 `HashMap`, `LinkedHashMap`, `TreeMap` 等,它们在底层数据结构和遍历性能上各有千秋。
以 `HashMap` 为例,它基于哈希表实现,内部维护了一个数组和链表的结合体(Java 8 之后引入了红黑树)。遍历 `HashMap` 时,需要特别关注键值对的顺序是不确定的。另一方面,`TreeMap` 基于红黑树实现,遍历时可以保证键的自然排序或者构造时提供的 `Comparator`。
示例代码块如下:
```java
import java.util.HashMap;
import java.util.Map;
public class Main {
public static void main(String[] args) {
Map<Integer, String> map = new HashMap<>();
map.put(1, "One");
map.put(2, "Two");
map.put(3, "Three");
for (Map.Entry<Integer, String> entry : map.entrySet()) {
System.out.println("Key: " + entry.getKey() + ", Value: " + entry.getValue());
}
}
}
```
此代码展示了如何通过 `entrySet()` 方法和增强型 for 循环遍历 `HashMap`。该方法允许我们在遍历过程中同时访问键和值。
#### 2.1.2 遍历Map的常见方式解析
遍历 Map 的方式主要有以下几种:
- 使用 `entrySet()` 遍历键值对。
- 使用 `keySet()` 只遍历键,再通过键获取值。
- 使用 `values()` 只遍历值。
- 使用迭代器 `Iterator` 遍历。
选择合适的方法取决于具体需求。例如,如果需要同时处理键和值,则推荐使用 `entrySet()`。
```java
// 通过keySet()遍历键
for(Integer key : map.keySet()) {
String value = map.get(key);
System.out.println("Key: " + key + ", Value: " + value);
}
// 通过values()遍历值
for(String value : map.values()) {
System.out.println("Value: " + value);
}
```
以上代码块展示了不同的遍历方式,每种方式各有优势。例如,`values()` 方法在只关心值时非常简洁高效。
### 2.2 遍历过程中性能考量
#### 2.2.1 时间复杂度与空间复杂度分析
遍历 Java Map 时的时间复杂度通常与 Map 的具体实现相关。例如,`HashMap` 和 `LinkedHashMap` 在最坏的情况下(所有键的哈希值相同)会退化到 O(n),而 `TreeMap` 基于红黑树,遍历的时间复杂度通常为 O(n log n)。
空间复杂度主要取决于键值对的大小。与时间复杂度类似,不同实现的空间复杂度也会有所不同。例如,`HashMap` 和 `LinkedHashMap` 需要额外的存储空间来处理哈希冲突,而 `TreeMap` 需要额外的空间来维持红黑树的平衡。
#### 2.2.2 高效遍历的性能对比
在进行 Map 遍历时,需要综合考虑各种因素。比如,如果需要频繁地进行排序操作,`TreeMap` 可能更适合,因为它的键天生就是排序的。如果需要更快的访问速度,`HashMap` 可能是更好的选择,尽管它不保证遍历的顺序。
具体性能测试方法可能包括创建不同大小的 Map,使用不同的遍历方式,然后通过计时来对比性能。需要注意的是,性能测试可能因为运行时环境、JVM 参数、垃圾回收等因素而产生差异。
### 2.3 遍历的正确性和异常处理
#### 2.3.1 避免ConcurrentModificationException
在多线程环境下或在遍历过程中对 Map 进行结构性修改(如添加或删除元素),可能会抛出 `ConcurrentModificationException` 异常。为了避免这种情况,可以使用迭代器的 `remove()` 方法进行安全的删除操作,或者在创建迭代器后不修改集合。
```java
Iterator<Map.Entry<Integer, String>> iterator = map.entrySet().iterator();
while (iterator.hasNext()) {
Map.Entry<Integer, String> entry = iterator.next();
if ("Two".equals(entry.getValue())) {
iterator.remove(); // 安全删除元素
}
}
```
此代码块演示了如何在遍历 Map 时安全地删除条目。
#### 2.3.2 空值处理与迭代器安全使用
处理空值是 Map 遍历中另一个需要关注的问题。在遍历过程中可能遇到的空值包括 `null` 键和 `null` 值。在使用迭代器时,可以通过 `hasNext()` 和 `next()` 方法检查并获取元素,这样即使 Map 中含有空值也不会抛出 `NullPointerException`。
```java
Iterator<Map.Entry<Integer, String>> iterator = map.entrySet().iterator();
while (iterator.hasNext()) {
Map.Entry<Integer, String> entry = iterator.next();
if (entry.getValue() == null) {
// 处理值为null的情况
System.out.println("Key: " + entry.getKey() + ", Value: NULL");
}
}
```
这个例子演示了如何检查并处理遍历过程中遇到的空值。
## 第三章:Java Map遍历的实用技巧
### 3.1 常规遍历技巧
#### 3.1.1 使用for-each循环遍历
for-each 循环(增强型 for 循环)是 Java 中遍历集合的简洁方式,可以用来遍历 Map 的 `entrySet`、`keySet` 或 `values`。这种方式尤其适合直接操作键值对或值。
```java
// 使用for-each循环遍历entrySet
for (Map.Entry<Integer, String> entry : map.entrySet()) {
Integer key = entry.getKey();
String value = entry.getValue();
// 对键和值进行操作
}
// 使用for-each循环遍历values
for (String value : map.values()) {
// 对值进行操作
}
```
### 3.2 高级遍历技术
#### 3.2.1 Lambda表达式与Stream API遍历
Java 8 引入了 Lambda 表达式和 Stream API,为 Map 遍历提供了更为灵活和强大的处理方式。Lambda 表达式使得代码更加简洁,而 Stream API 则提供了强大的数据处理能力。
```java
// 使用Stream API和Lambda表达式遍历Map
map.entrySet().stream()
.filter(entry -> "One".equals(entry.getValue())) // 过滤条件
.forEach(entry -> System.out.println("Filtered Value: " + entry.getValue()));
```
该代码块展示了如何使用 Stream API 对 Map 进行过滤并遍历。在处理复杂的数据处理逻辑时,Stream API 可以提供更多的灵活性和强大的操作能力。
#### 3.2.2 使用Java 8的forEach方法
`forEach` 是 Stream API 中的一个方
0
0
相关推荐
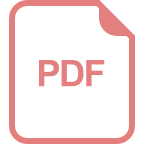
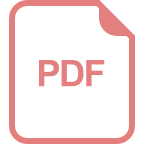
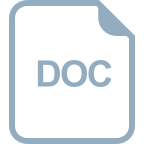
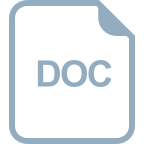
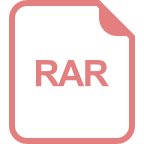
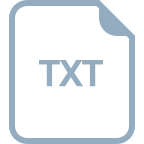
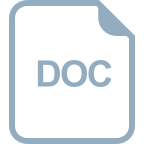
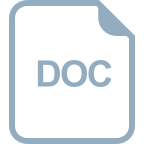