使用C语言创建具备增删改查功能的通讯录链表,关键地方加入注释尽可能通俗易懂
时间: 2024-04-29 07:22:43 浏览: 73
以下是使用C语言创建通讯录链表的示例代码,包括增删改查四个功能。代码中注释尽可能地解释了关键地方的作用,希望对您有所帮助。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义通讯录结构体
typedef struct Contact {
char name[20];
char phone[20];
struct Contact *next;
} Contact;
// 创建通讯录链表
Contact *create() {
Contact *head = (Contact *)malloc(sizeof(Contact));
head->next = NULL;
return head;
}
// 添加联系人
void add(Contact *head) {
Contact *p = head;
while (p->next != NULL) { // 遍历链表,找到最后一个节点
p = p->next;
}
Contact *new_contact = (Contact *)malloc(sizeof(Contact)); // 新建节点
printf("请输入姓名和电话(用空格分隔):");
scanf("%s %s", new_contact->name, new_contact->phone);
new_contact->next = NULL;
p->next = new_contact; // 将新节点插入链表尾部
printf("联系人添加成功!\n");
}
// 删除联系人
void del(Contact *head) {
char name[20];
printf("请输入要删除的联系人姓名:");
scanf("%s", name);
Contact *p = head->next;
Contact *pre = head; // pre为p的前一个节点
while (p != NULL) {
if (strcmp(p->name, name) == 0) { // 找到要删除的联系人
pre->next = p->next; // 将p从链表中删除
free(p);
printf("联系人删除成功!\n");
return;
}
pre = p;
p = p->next;
}
printf("未找到要删除的联系人!\n");
}
// 修改联系人
void modify(Contact *head) {
char name[20];
printf("请输入要修改的联系人姓名:");
scanf("%s", name);
Contact *p = head->next;
while (p != NULL) {
if (strcmp(p->name, name) == 0) { // 找到要修改的联系人
printf("请输入新的姓名和电话(用空格分隔):");
scanf("%s %s", p->name, p->phone);
printf("联系人修改成功!\n");
return;
}
p = p->next;
}
printf("未找到要修改的联系人!\n");
}
// 查找联系人
void search(Contact *head) {
char name[20];
printf("请输入要查找的联系人姓名:");
scanf("%s", name);
Contact *p = head->next;
while (p != NULL) {
if (strcmp(p->name, name) == 0) { // 找到要查找的联系人
printf("姓名:%s,电话:%s\n", p->name, p->phone);
return;
}
p = p->next;
}
printf("未找到要查找的联系人!\n");
}
// 主函数
int main() {
Contact *head = create(); // 创建通讯录链表
int choice;
while (1) {
printf("\n请选择操作:\n1.添加联系人\n2.删除联系人\n3.修改联系人\n4.查找联系人\n5.退出\n");
scanf("%d", &choice);
switch (choice) {
case 1:
add(head);
break;
case 2:
del(head);
break;
case 3:
modify(head);
break;
case 4:
search(head);
break;
case 5:
exit(0);
default:
printf("输入有误,请重新输入!\n");
break;
}
}
return 0;
}
```
阅读全文
相关推荐
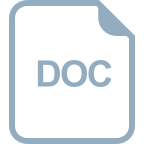
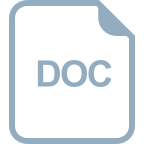
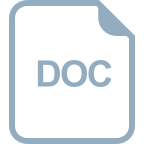
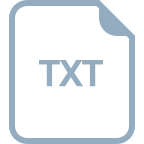
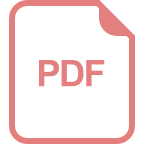
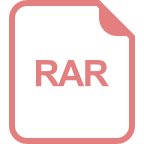
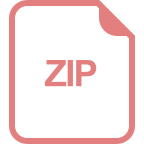
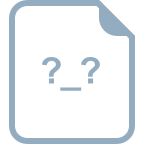
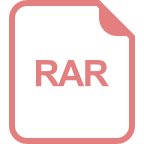
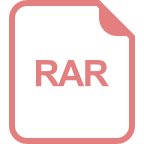
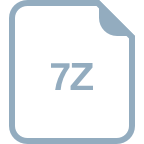
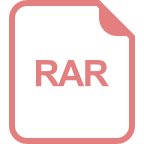
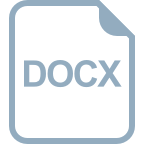