用c++构造一个日期类Date,要求重载:流提取和流插入运算符,重载前置和后置自增运算符(必要时递增年月)。类定义如下: // Date class definition. #ifndef DATE_H #define DATE_H #include <iostream> using std::ostream; class Date { friend ostream &operator<<( ostream &, const Date & ); public: Date( int m = 1, int d = 1, int y = 1900 ); // default constructor void setDate( int, int, int ); // set month, day, year Date &operator++(); // prefix increment operator Date operator++( int ); // postfix increment operator const Date &operator+=( int ); // add days, modify object bool leapYear( int ) const; // is date in a leap year? bool endOfMonth( int ) const; // is date at the end of month? private: int month; int day; int year; static const int days[]; // array of days per month void helpIncrement(); // utility function for incrementing date }; // end class Date #endif
时间: 2024-01-06 11:04:13 浏览: 127
以下是基于你提供的类定义的C++代码:
```
#include <iostream>
#include "Date.h"
using std::ostream;
// Define static member days.
const int Date::days[] = { 0, 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31 };
// Constructor: initialize month, day, year.
Date::Date(int m, int d, int y)
{
setDate(m, d, y);
}
// Set the date.
void Date::setDate(int m, int d, int y)
{
month = (m >= 1 && m <= 12) ? m : 1;
year = (y >= 1900 && y <= 2100) ? y : 1900;
// Test for a leap year.
if (month == 2 && leapYear(year))
day = (d >= 1 && d <= 29) ? d : 1;
else
day = (d >= 1 && d <= days[month]) ? d : 1;
}
// Prefix increment operator.
Date& Date::operator++()
{
helpIncrement();
return *this;
}
// Postfix increment operator.
Date Date::operator++(int)
{
Date temp = *this;
helpIncrement();
return temp;
}
// Add days to the date.
const Date& Date::operator+=(int additionalDays)
{
for (int i = 0; i < additionalDays; i++)
helpIncrement();
return *this;
}
// Determine if the year is a leap year.
bool Date::leapYear(int testYear) const
{
if (testYear % 400 == 0 || (testYear % 100 != 0 && testYear % 4 == 0))
return true;
else
return false;
}
// Determine if the date is at the end of the month.
bool Date::endOfMonth(int testDay) const
{
if (month == 2 && leapYear(year))
return testDay == 29;
else
return testDay == days[month];
}
// Utility function to increment the date.
void Date::helpIncrement()
{
if (!endOfMonth(day))
day++;
else
{
if (month < 12)
month++;
else
{
month = 1;
year++;
}
day = 1;
}
}
// Overloaded stream insertion operator.
ostream& operator<<(ostream& output, const Date& d)
{
static char* monthName[13] = { "", "January", "February",
"March", "April", "May", "June", "July", "August", "September",
"October", "November", "December" };
output << monthName[d.month] << ' ' << d.day << ", " << d.year;
return output;
}
```
可以在代码中添加其他函数和运算符的实现以满足你的需求。
阅读全文
相关推荐
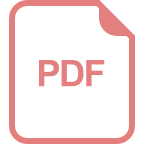
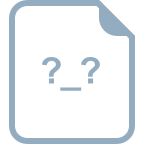
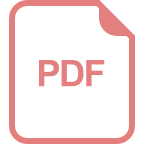
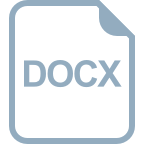
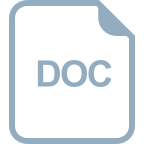
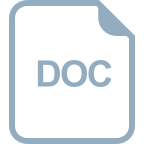
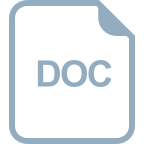
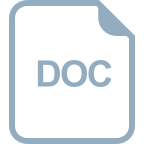
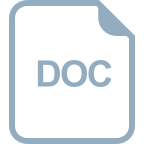
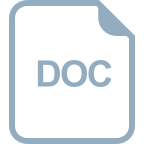
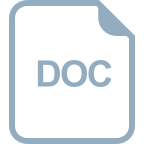
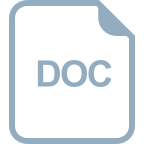





