torch 变onehot 固定维度
时间: 2023-07-19 18:11:01 浏览: 86
如果需要将整数张量转换为固定维度的one-hot编码形式的张量,可以使用`torch.nn.functional.one_hot()`函数的`num_classes`参数来指定one-hot编码的维度。如果输入的整数张量中存在超过`num_classes`的值,则会抛出异常。下面是一个示例代码:
```
import torch
# 定义一个整数张量
int_tensor = torch.tensor([1, 2, 0, 1])
# 将整数张量转换为3维的one-hot编码形式的张量
one_hot_tensor = torch.nn.functional.one_hot(int_tensor, num_classes=3)
print(one_hot_tensor)
```
输出结果:
```
tensor([[0, 1, 0],
[0, 0, 1],
[1, 0, 0],
[0, 1, 0]])
```
其中,`num_classes`参数指定了one-hot编码的维度为3。由于输入的整数张量的元素值不超过3,因此输出的one-hot编码形式的张量的每行都是3维的。
相关问题
torch 变onehot
可以使用PyTorch中的函数`torch.nn.functional.one_hot()`将一个整数张量转换为one-hot编码形式的张量。下面是一个示例代码:
```
import torch
# 定义一个整数张量
int_tensor = torch.tensor([1, 2, 0, 1])
# 将整数张量转换为one-hot编码形式的张量
one_hot_tensor = torch.nn.functional.one_hot(int_tensor)
print(one_hot_tensor)
```
输出结果:
```
tensor([[0, 1, 0],
[0, 0, 1],
[1, 0, 0],
[0, 1, 0]])
```
其中,输入的整数张量的每个元素值表示对应的one-hot编码中为1的位置的索引,而输出的one-hot编码形式的张量的每行表示对应的整数张量元素的one-hot编码。
torch mask tensor onehot
在PyTorch中,可以通过使用torch的函数和方法来进行掩码(mask)和独热编码(one-hot encoding)的操作。
对于掩码操作,可以使用torch的逻辑运算符(如torch.lt,torch.gt,torch.eq)生成一个与原始张量具有相同形状的布尔型张量。布尔型掩码张量中,True表示满足某个条件,而False表示不满足。可以将掩码张量应用于其他张量,根据掩码张量中元素的True或False值选择需要操作的元素。例如:
``` python
import torch
x = torch.tensor([1, 2, 3, 4, 5])
mask = torch.lt(x, 3) # 创建掩码张量(小于3的元素)
masked_x = x[mask] # 应用掩码张量来获取满足条件的元素
print(masked_x)
```
对于独热编码操作,可以使用torch的函数one_hot。该函数接受一个整数张量和一个类别数作为输入,并返回一个具有相同形状但包含独热向量的张量。每个独热向量对应于输入张量中对应位置的值。例如:
``` python
import torch
x = torch.tensor([2, 1, 0])
one_hot_x = torch.nn.functional.one_hot(x, num_classes=3) # 进行独热编码
print(one_hot_x)
```
以上是在PyTorch中进行掩码和独热编码的基本操作。根据具体应用的需求和使用场景,可以进一步调整和扩展这些操作。
阅读全文
相关推荐
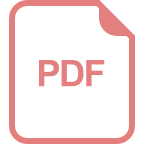
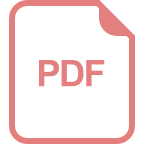
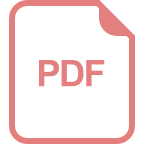













