用C语言设计一个算法,利用顺序栈的基本运算 从栈顶到栈底输出栈中的所有元素,要求仍保持栈中元素不变。
时间: 2023-06-15 22:08:00 浏览: 107
以下是基于顺序栈的算法实现:
```c
#include <stdio.h>
#define MAXSIZE 100
typedef struct {
int data[MAXSIZE];
int top;
} SqStack;
void InitStack(SqStack *S) {
S->top = -1;
}
int Push(SqStack *S, int x) {
if (S->top == MAXSIZE-1) {
return 0; // 栈满,插入失败
}
S->top++;
S->data[S->top] = x;
return 1; // 插入成功
}
int Pop(SqStack *S, int *x) {
if (S->top == -1) {
return 0; // 栈空,弹出失败
}
*x = S->data[S->top];
S->top--;
return 1; // 弹出成功
}
void PrintStack(SqStack S) {
int i;
printf("Stack elements from top to bottom: ");
for (i = S.top; i >= 0; i--) {
printf("%d ", S.data[i]);
}
printf("\n");
}
int main() {
SqStack S;
int i, x;
InitStack(&S);
for (i = 0; i < 5; i++) {
Push(&S, i+1);
}
PrintStack(S);
for (i = S.top; i >= 0; i--) {
Pop(&S, &x);
Push(&S, x);
}
PrintStack(S);
return 0;
}
```
该算法通过弹出栈顶元素并将其保存在一个临时变量中,再将该临时变量重新插入栈底,从而实现了从栈顶到栈底输出栈中的所有元素的要求,同时保持了栈中元素不变。
相关推荐
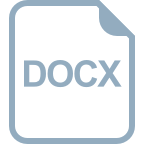
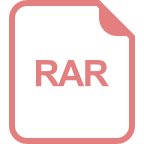
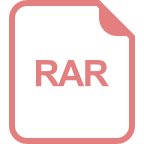












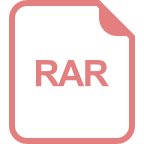
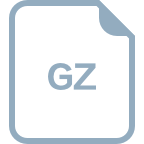
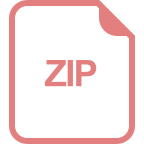