C#高效的Bayer近邻插值源码
时间: 2023-10-19 18:23:58 浏览: 209
以下是C#实现的Bayer近邻插值算法的示例代码:
```csharp
public static void BayerInterpolation(byte[] input, int width, int height, byte[] output)
{
int stride = width * 3;
int r, g, b;
for (int i = 1; i < height - 1; i += 2)
{
for (int j = 1; j < width - 1; j += 2)
{
// Green pixel
g = input[i * width + j];
if (i > 1 && j > 1 && i < height - 2 && j < width - 2)
{
// Use all neighbors
r = (input[(i - 1) * width + j] + input[(i + 1) * width + j] + input[i * width + j - 1] + input[i * width + j + 1]) / 4;
b = (input[(i - 1) * width + j - 1] + input[(i - 1) * width + j + 1] + input[(i + 1) * width + j - 1] + input[(i + 1) * width + j + 1]) / 4;
}
else if (i == 1 && j == 1)
{
// Top-left corner
r = (input[i * width + j + 1] + input[(i + 1) * width + j]) / 2;
b = input[(i + 1) * width + j + 1];
}
else if (i == 1 && j == width - 2)
{
// Top-right corner
r = (input[i * width + j - 1] + input[(i + 1) * width + j]) / 2;
b = input[(i + 1) * width + j - 1];
}
else if (i == height - 2 && j == 1)
{
// Bottom-left corner
r = (input[i * width + j + 1] + input[(i - 1) * width + j]) / 2;
b = input[(i - 1) * width + j + 1];
}
else if (i == height - 2 && j == width - 2)
{
// Bottom-right corner
r = (input[i * width + j - 1] + input[(i - 1) * width + j]) / 2;
b = input[(i - 1) * width + j - 1];
}
else if (i == 1)
{
// Top row
r = (input[i * width + j - 1] + input[i * width + j + 1] + input[(i + 1) * width + j]) / 3;
b = (input[(i + 1) * width + j - 1] + input[(i + 1) * width + j + 1]) / 2;
}
else if (i == height - 2)
{
// Bottom row
r = (input[i * width + j - 1] + input[i * width + j + 1] + input[(i - 1) * width + j]) / 3;
b = (input[(i - 1) * width + j - 1] + input[(i - 1) * width + j + 1]) / 2;
}
else if (j == 1)
{
// Left column
r = (input[(i - 1) * width + j] + input[(i + 1) * width + j] + input[i * width + j + 1]) / 3;
b = (input[(i - 1) * width + j + 1] + input[(i + 1) * width + j + 1]) / 2;
}
else
{
// Right column
r = (input[(i - 1) * width + j] + input[(i + 1) * width + j] + input[i * width + j - 1]) / 3;
b = (input[(i - 1) * width + j - 1] + input[(i + 1) * width + j - 1]) / 2;
}
output[(i * width + j) * 3] = (byte)b;
output[(i * width + j) * 3 + 1] = (byte)g;
output[(i * width + j) * 3 + 2] = (byte)r;
}
}
}
```
其中,`input`是原始的Bayer图像数据,`width`和`height`是图像的宽度和高度,`output`是插值后的RGB图像数据。该算法采用了近邻插值的方法,对每个绿色像素进行插值,然后计算出对应的红色和蓝色像素的值。根据不同的位置,采用不同的近邻像素进行插值。最后将插值后的RGB像素值写入`output`数组中。
阅读全文
相关推荐
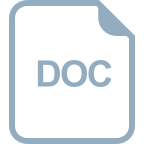
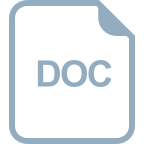
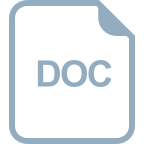
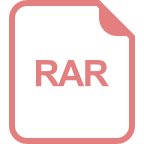
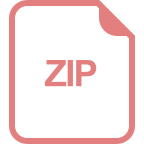


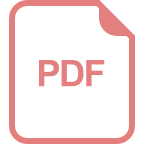
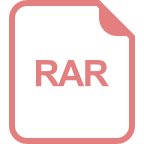








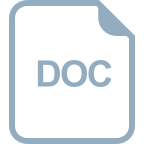
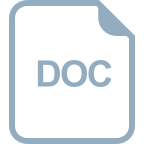