def getMediaInfo(self, media_id): resp = self.request('https://pacaio.match.qq.com/om/detailInfo?id={}&_time={}' . format(media_id, int(time.time() * 1000)), 'GET', {}) if not resp: print('请求数据失败,请检查你的网络环境') return '' return json.loads(resp)['data']
时间: 2023-09-16 15:16:06 浏览: 43
这段代码是该类的一个方法,用于获取指定媒体id的详细信息,参数media_id表示媒体的id。方法内部首先使用request方法向指定的URL发送GET请求,获取媒体的JSON数据。其中,请求URL是通过格式化字符串的方式构建而成,包含了当前时间的时间戳,可以保证URL的唯一性。如果请求失败,则在控制台输出错误信息并返回空字符串。如果请求成功,则将返回的JSON数据使用json模块的loads方法转换为Python对象(dict或list)并返回其中的"data"字段。注意,在获取媒体信息时,没有使用xpath或正则表达式等方式解析HTML页面,而是直接获取JSON数据,这种方式通常更加高效。
相关问题
import requests from bs4 import BeautifulSoup import openpyxl class LianJiaSpider(): def __init__(self): self.url = 'https://bj.lianjia.com/ershoufang/pg{0}/' self.headers = {'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; WOW64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/92.0.4515.131 Safari/537.36 SLBrowser/8.0.0.12022 SLBChan/109'} def send_request(self, url): resp = requests.get(url, headers=self.headers) if resp.status_code == 200: return resp def parse_html(self, resp): lst = [] html = resp.text bs = BeautifulSoup(html, 'lxml') ul = bs.find('ul', class_='sellListContent') li_list = ul.find_all('li') for item in li_list: title = item.find('div', class_='title').text positionInfo = item.find('div', class_='positionInfo').text address = item.find('div', class_='address').text followInfo = item.find('div', class_='followInfo').text tag = item.find('div', class_='tag').text totalPrice = item.find('div', class_='totalPrice totalPrice2').text unitPrice = item.find('div', class_='unitPrice').text # print(unitPrice) lst.append((title, positionInfo, address, followInfo, tag, totalPrice, unitPrice)) print(lst) self.save(lst) def save(self, lst): wb = openpyxl.Workbook() sheet = wb.active for row in lst: sheet.append(row) continue wb.save('D:/爬虫/链家.csv') def start(self): for i in range(1, 5): full_url = self.url.format(i) resp = self.send_request(full_url) #print(resp.text) self.parse_html(resp) if __name__ == '__main__': lianjia = LianJiaSpider() lianjia.start()使用以上代码爬取数据保存到文件中只显示最后一页30条数据,前面页码的数据都被覆盖了,如何更改
在 `save` 方法中,每次循环都重新创建了一个新的 `Workbook` 对象,因此在保存数据时会覆盖之前的数据。您可以将 `Workbook` 对象的创建放在 `__init__` 方法中,这样每次保存数据时都可以使用同一个 `Workbook` 对象。另外,您还可以将文件名中的页码部分替换为变量 `i`,这样每次保存的文件都会有不同的文件名,不会被覆盖。修改后的代码如下:
```python
import requests
from bs4 import BeautifulSoup
import openpyxl
class LianJiaSpider():
def __init__(self):
self.url = 'https://bj.lianjia.com/ershoufang/pg{0}/'
self.headers = {'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; WOW64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/92.0.4515.131 Safari/537.36 SLBrowser/8.0.0.12022 SLBChan/109'}
self.wb = openpyxl.Workbook()
self.sheet = self.wb.active
self.sheet.append(('标题', '位置', '地址', '关注信息', '标签', '总价', '单价'))
def send_request(self, url):
resp = requests.get(url, headers=self.headers)
if resp.status_code == 200:
return resp
def parse_html(self, resp):
html = resp.text
bs = BeautifulSoup(html, 'lxml')
ul = bs.find('ul', class_='sellListContent')
li_list = ul.find_all('li')
for item in li_list:
title = item.find('div', class_='title').text
positionInfo = item.find('div', class_='positionInfo').text
address = item.find('div', class_='address').text
followInfo = item.find('div', class_='followInfo').text
tag = item.find('div', class_='tag').text
totalPrice = item.find('div', class_='totalPrice totalPrice2').text
unitPrice = item.find('div', class_='unitPrice').text
self.sheet.append((title, positionInfo, address, followInfo, tag, totalPrice, unitPrice))
print('第{}页数据爬取完成'.format(i))
def save(self):
self.wb.save('D:/爬虫/链家_{}.csv'.format(i))
def start(self):
for i in range(1, 5):
full_url = self.url.format(i)
resp = self.send_request(full_url)
self.parse_html(resp)
self.save()
if __name__ == '__main__':
lianjia = LianJiaSpider()
lianjia.start()
```
在修改后的代码中,我将文件名改为了 `"D:/爬虫/链家_{}.csv".format(i)`,这样每次保存的文件名都会包含页码,不会被覆盖。同时,我将保存数据的操作从 `parse_html` 方法中分离出来,放在了单独的 `save` 方法中,并且在 `__init__` 方法中创建了 `Workbook` 对象和表头。这样就可以避免每次循环都重新创建一个新的 `Workbook` 对象,从而避免了数据被覆盖的问题。
解释代码: def __init__(self, X, n_components, start_df, random_state, init_type): self.loc_, self.scale_, self.scale_inv_chole_, self.scale_chole_ = \ self.initialize_params(X, n_components, random_state, init_type) self.df_ = np.full(shape=n_components, fill_value=start_df) self.resp = None self.a_nm, self.b_nm = None, None self.E_gamma = None self.E_log_gamma = None self.E_log_weights = None self.wishart_vm = None self.kappa_m = None self.eta_m = None
这段代码定义了一个 Python 类的初始化方法 `__init__`。这个方法接收参数 `X`,`n_components`,`start_df`,`random_state` 和 `init_type`。
初始化方法会使用 `initialize_params` 方法对 `self.loc_`,`self.scale_`,`self.scale_inv_chole_` 和 `self.scale_chole_` 进行赋值。
然后,`self.df_` 将使用 `np.full` 方法创建一个 shape 为 `n_components`,元素都是 `start_df` 的数组。
其他变量 `self.resp`,`self.a_nm`,`self.b_nm`,`self.E_gamma`,`self.E_log_gamma`,`self.E_log_weights`,`self.wishart_vm`,`self.kappa_m` 和 `self.eta_m` 都被初始化为 `None`。
相关推荐
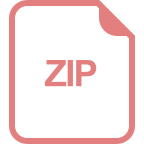













