如何在Python中实现模拟退火算法以优化组合问题,并提供一个代码示例来解释其工作原理?
时间: 2024-12-05 18:27:55 浏览: 26
模拟退火算法是一种启发式搜索算法,适用于解决组合优化问题,比如旅行商问题(TSP)、背包问题等。要在Python中实现模拟退火算法,你需要定义一个适应度函数来衡量解的优劣,并通过温度控制策略来引导搜索过程。以下是一个简化版的代码示例,用于解决TSP问题:
参考资源链接:[掌握模拟退火算法:Python实现详解](https://wenku.csdn.net/doc/7bv17fqr87?spm=1055.2569.3001.10343)
```python
import math
import random
# 计算两点间的欧几里得距离
def euclidean_distance(point1, point2):
return math.sqrt((point1[0] - point2[0])**2 + (point1[1] - point2[1])**2)
# 计算路径长度,即总距离
def calculate_total_distance(points, route):
total_distance = 0
for i in range(len(route)):
total_distance += euclidean_distance(points[route[i-1]], points[route[i]])
return total_distance
# 生成新解(邻域解)
def generate_new_route(current_route):
new_route = current_route[:]
l = len(new_route)
i, j = random.sample(range(l), 2)
new_route[i], new_route[j] = new_route[j], new_route[i]
return new_route
# 模拟退火算法实现
def simulated_annealing(points, temperature, cooling_rate, stopping_temperature):
current_route = list(range(len(points)))
current_distance = calculate_total_distance(points, current_route)
best_route = current_route[:]
best_distance = current_distance
while temperature > stopping_temperature:
new_route = generate_new_route(current_route)
new_distance = calculate_total_distance(points, new_route)
# 接受准则,使用Metropolis准则
if new_distance < current_distance or random.random() < math.exp((current_distance - new_distance) / temperature):
current_route = new_route
current_distance = new_distance
if new_distance < best_distance:
best_route = new_route
best_distance = new_distance
temperature *= (1 - cooling_rate)
return best_route, best_distance
# 定义一组城市的坐标点
cities = [(0, 0), (1, 5), (2, 2), (3, 8), (4, 4)]
# 算法参数设置
initial_temperature = 1000
cooling_rate = 0.003
stopping_temperature = 1e-8
# 执行模拟退火算法
best_route, best_distance = simulated_annealing(cities, initial_temperature, cooling_rate, stopping_temperature)
print(
参考资源链接:[掌握模拟退火算法:Python实现详解](https://wenku.csdn.net/doc/7bv17fqr87?spm=1055.2569.3001.10343)
阅读全文
相关推荐
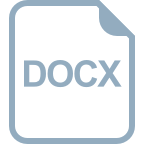
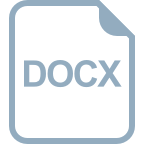
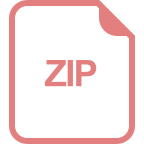



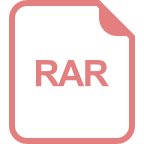
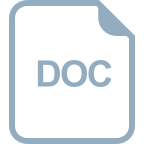
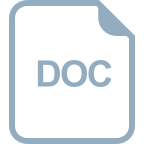
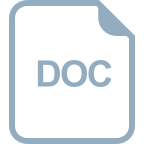
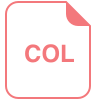
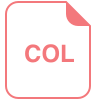
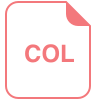
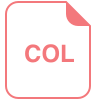
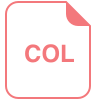
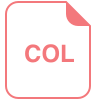


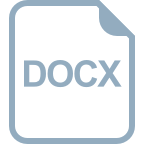