模拟退火算法python
时间: 2023-09-15 21:05:17 浏览: 69
### 回答1:
模拟退火算法(Simulated Annealing,SA)是一种全局优化算法,常用于解决各种复杂的组合优化问题。下面是一个基本的模拟退火算法的 Python 实现:
```python
import random
import math
# 目标函数
def objective_function(x):
return math.sin(x)
# 初始解
def initial_solution():
return random.uniform(-math.pi, math.pi)
# 定义温度函数
def temperature(k, T0):
alpha = 0.95
return T0 * (alpha ** k)
# 定义状态产生函数
def neighbor(x, delta):
return x + random.uniform(-delta, delta)
# 定义接受准则
def acceptance_criterion(delta, temperature):
if delta < 0:
return True
else:
p = math.exp(-delta / temperature)
return random.random() < p
# 模拟退火算法
def simulated_annealing(kmax, T0, delta):
# 初始化当前解和最优解
x_curr = initial_solution()
x_best = x_curr
# 迭代过程
for k in range(kmax):
# 产生新的状态
x_new = neighbor(x_curr, delta)
# 计算能量差
delta_E = objective_function(x_new) - objective_function(x_curr)
# 如果新状态更优,则接受
if acceptance_criterion(delta_E, temperature(k, T0)):
x_curr = x_new
# 更新最优解
if objective_function(x_curr) < objective_function(x_best):
x_best = x_curr
return x_best
# 测试
x_best = simulated_annealing(kmax=1000, T0=100, delta=0.5)
print("最优解:", x_best)
print("最优值:", objective_function(x_best))
```
这个实现中,目标函数是 `math.sin(x)`,初始解是在区间 `[-π, π]` 内随机生成的,温度函数为 $T_k = T_0 \times \alpha^k$,其中 $\alpha = 0.95$,状态产生函数是在当前解的基础上,加上一个在 $[-\delta, \delta]$ 内随机产生的扰动,接受准则使用了 Boltzmann 分布的形式,即 $\mathrm{P}(\Delta E) = \exp(-\Delta E/T)$。在每次迭代中,首先产生一个新状态,然后计算能量差 $\Delta E$,如果新状态更优,则接受。最后,输出最优解和最优值。
需要注意的是,模拟退火算法的结果可能受到很多参数的影响,比如初始温度 $T_0$、温度下降速率 $\alpha$、状态产生函数的扰动范围 $\delta$ 等等,需要根据具体问题进行调整。
### 回答2:
模拟退火算法是一种基于概率的全局优化算法,常用于求解复杂问题。
简单来说,模拟退火算法通过模拟固体退火的过程来逐渐降低系统能量,从而找到最优解。其基本思路是通过在初始解附近进行随机搜索,并接受部分劣解,从而有机会跳出当前局部最优解并找到潜在的全局最优解。
使用Python实现模拟退火算法的基本步骤如下:
1. 初始化:定义问题的初始解、温度和冷却率。
2. 外循环:反复迭代,直到满足终止条件(例如温度降至某个阈值或达到最大迭代次数)。
3. 内循环:在当前温度下,进行随机扰动并得到新的解。
4. 接受准则:根据一定的概率接受新解,一般采用Metropolis准则:若新解优于当前解,则直接接受;否则以一定概率接受差解,概率与温度和差解程度有关。
5. 更新温度:根据设定的冷却率逐渐降低温度。
6. 返回最优解。
以下是一个基本的模拟退火算法的Python实现示例:
```python
import random
import math
def simulated_annealing(initial_solution, initial_temperature, cooling_rate):
current_solution = initial_solution
best_solution = current_solution
temperature = initial_temperature
while temperature > 1:
for i in range(cooling_rate):
new_solution = get_neighbor(current_solution)
energy_delta = calculate_energy(new_solution) - calculate_energy(current_solution)
if energy_delta < 0 or random.random() < math.exp(-energy_delta / temperature):
current_solution = new_solution
if calculate_energy(current_solution) < calculate_energy(best_solution):
best_solution = current_solution
temperature *= cooling_rate
return best_solution
def get_neighbor(solution):
# 实现获取相邻解的逻辑
pass
def calculate_energy(solution):
# 实现计算解的能量的逻辑
pass
# 调用示例
initial_solution = ...
initial_temperature = ...
cooling_rate = ...
best_solution = simulated_annealing(initial_solution, initial_temperature, cooling_rate)
print(best_solution)
```
在实际应用中,需要根据具体问题定义相邻解的生成方法和能量计算方法,并根据问题特性调整初始温度和冷却率等参数,以获得更好的求解效果。
### 回答3:
模拟退火算法(Simulated Annealing)是一种启发式优化算法,常用于求解最优化问题。该算法的基本思想源于固体退火原理,通过模拟物质退火过程中的冷却过程,以一定的概率接受劣质解,从而避免陷入局部最优解,寻找全局最优解。
以下是使用Python实现模拟退火算法的步骤:
1. 初始化当前解和初始解温度:
- 将当前解设为初始解。
- 设置初始解温度。
2. 迭代更新当前解:
- 在当前解的邻域中生成一个新解。
- 计算新解的目标函数值与当前解的目标函数值的差值。
- 如果新解的目标函数值较好,则接受新解作为当前解。
- 如果新解的目标函数值较差,则以一定的概率接受新解作为当前解(概率公式通常为exp(-delta/T),其中delta为目标函数值差值,T为当前解温度)。
- 更新当前解温度。
3. 判断停止条件:
- 当达到停止条件(如迭代次数、目标函数值变化很小等)时,停止迭代并输出结果。
- 否则,返回步骤2继续迭代更新当前解。
通过以上步骤,我们可以实现一个基本的模拟退火算法。
代码示例:
```python
import math
import random
def simulated_annealing():
# 初始化当前解和初始解温度
current_solution = initial_solution()
current_temp = initial_temperature()
while not stop_condition():
# 在当前解的邻域中生成一个新解
new_solution = get_neighbor(current_solution)
# 计算新解的目标函数值与当前解的目标函数值的差值
delta = calculate_delta(current_solution, new_solution)
# 如果新解的目标函数值较好,则接受新解作为当前解
if delta > 0:
current_solution = new_solution
else:
# 如果新解的目标函数值较差,则以一定的概率接受新解作为当前解
accept_prob = math.exp(delta / current_temp)
if random.random() < accept_prob:
current_solution = new_solution
# 更新当前解温度
current_temp = update_temperature(current_temp)
return current_solution
# 根据具体问题定义初始化当前解和初始解温度的函数
def initial_solution():
# 初始化当前解
pass
def initial_temperature():
# 初始化初始解温度
pass
# 根据具体问题定义获取当前解邻域的函数
def get_neighbor(current_solution):
# 获取当前解的邻域中的一个新解
pass
# 根据具体问题定义计算目标函数值差值的函数
def calculate_delta(current_solution, new_solution):
# 计算新解的目标函数值与当前解的目标函数值的差值
pass
# 根据具体问题定义停止条件的函数
def stop_condition():
# 判断是否满足停止条件
pass
# 根据具体问题定义更新当前解温度的函数
def update_temperature(current_temp):
# 更新当前解温度
pass
```
以上是使用Python实现模拟退火算法的基本步骤,具体在每个函数中需要根据实际问题做进一步的定义和实现。
相关推荐
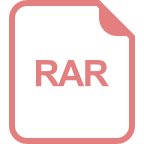














