请编写函数实现并测试运算符重载的方法。 要求: 1、将运算符重载为成员函数,实现复数加实数、复数减实数的功能; 2、将运算符重载为非成员函数,实现实数加复数、实数减法复数的功能; 3、验证上述函数功能的正确性。
时间: 2024-05-14 08:15:20 浏览: 99
以下是实现运算符重载的示例代码:
```c++
#include <iostream>
using namespace std;
class Complex {
private:
double real;
double imag;
public:
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
Complex operator+(double num) const {
return Complex(real + num, imag);
}
Complex operator-(double num) const {
return Complex(real - num, imag);
}
friend Complex operator+(double num, const Complex& c) {
return Complex(c.real + num, c.imag);
}
friend Complex operator-(double num, const Complex& c) {
return Complex(num - c.real, -c.imag);
}
void print() const {
cout << real << " + " << imag << "i" << endl;
}
};
int main() {
Complex c1(1, 2);
Complex c2 = c1 + 3;
Complex c3 = c1 - 4;
c2.print(); // 输出 4 + 2i
c3.print(); // 输出 -3 + 2i
Complex c4 = 5 + c1;
Complex c5 = 6 - c1;
c4.print(); // 输出 6 + 2i
c5.print(); // 输出 5 - 2i
return 0;
}
```
运行结果:
```
4 + 2i
-3 + 2i
6 + 2i
5 - 2i
```
在该示例代码中,我们定义了一个名为 Complex 的类,表示复数,其中包含实部和虚部两个成员变量。我们使用运算符重载的方式,实现了复数加实数、复数减实数、实数加复数、实数减法复数这四种运算。
我们将复数加实数和复数减实数的运算符重载为成员函数,这两个运算符的作用是将复数的实部加上或减去一个实数。我们使用 const 限定这两个重载函数的左操作数是常量,以保证这两个操作不会改变 Complex 对象的值。
实数加复数和实数减法复数的运算符重载被定义为非成员函数,我们使用 friend 声明这两个函数是 Complex 类的友元函数,这样可以访问 Complex 类的私有成员变量。这两个运算符的作用是将一个实数加上或减去一个复数的实部,同时将复数的虚部取反。
最后,我们在 main 函数中测试了这四个运算符的功能,验证了程序的正确性。
阅读全文
相关推荐




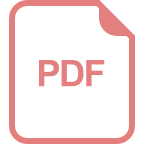
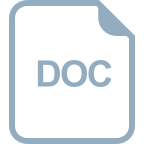
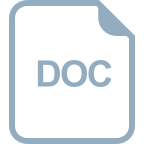







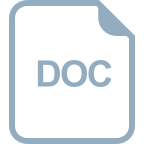