使用numpy设计一个softmax,要求随机生成一个10*10的随机数组,最后输出[1. 1. 1. 1. 1. 1. 1. 1. 1. 1.]
时间: 2024-09-27 10:18:16 浏览: 38
在Python中,我们可以使用NumPy库来实现softmax函数,它通常用于将一组数值转换成概率分布。首先,我们需要生成一个10x10的随机数组,然后对每个元素应用softmax操作。softmax函数公式是对于给定的一维向量z_i,其值为z_i / sum(z_j)。
以下是一个简单的步骤:
```python
import numpy as np
# 首先,生成一个10x10的随机数组
input_array = np.random.rand(10, 10)
# 对于每一行进行softmax计算
softmax_array = np.exp(input_array) / np.sum(np.exp(input_array), axis=1, keepdims=True)
# 输出结果应该接近于 [1. 1. 1. ... 1.]
print("Softmax array:")
print(softmax_array)
```
在这个例子中,`np.exp()`函数用于指数运算,`np.sum()`用于沿指定轴求和,并通过`keepdims=True`保持原始形状以便于后续除法。注意,softmax函数的结果总和会趋向于1,所以如果输出接近于`[1. 1. 1. ... 1.]`,那意味着数组已经经过了有效的softmax处理。
相关问题
import numpy as np def softmax(x): """ 计算softmax函数 """ exp_x = np.exp(x) return exp_x / np.sum(exp_x, axis=1, keepdims=True) # 生成随机输入数据,其中m为样本数,n为特征数,k为类别数 m, n, k = 10, 5, 3 x = np.random.randn(m, n) # 生成随机权重和偏置项 W = np.random.randn(n, k) b = np.random.randn(1, k) # 计算线性输出 z = np.dot(x, W) + b # 计算softmax输出 y_hat = softmax(z) print(y_hat)这段代码的输出是什么样的,具体解释一下
这段代码的输出是一个形状为(m, k)的二维数组,其中m为样本数,k为类别数。数组的每个元素表示对应样本属于每一类别的概率。具体解释如下:
首先,生成了一个形状为(m, n)的随机输入数据x,其中m为样本数,n为特征数,表示有m个样本,每个样本有n个特征。
然后,生成了形状为(n, k)的随机权重W和形状为(1, k)的随机偏置项b,其中k为类别数,表示共有k个类别。
接着,通过矩阵乘法计算出线性输出z,即z = x * W + b,其中*表示矩阵乘法。
最后,将线性输出z作为输入,经过softmax函数计算出每个样本属于每个类别的概率,即y_hat = softmax(z)。其中,softmax函数的作用是将原始输出z通过指数函数进行归一化,使得输出值在(0,1)之间,并且所有输出值之和为1。具体计算方法是先对z中每个元素进行指数运算,然后将每个样本的指数和作为分母,将每个样本的指数除以分母得到每个样本属于每个类别的概率。最后输出y_hat即为每个样本属于每个类别的概率。
以下代码出现input depth must be evenly divisible by filter depth: 1 vs 3错误是为什么,代码应该怎么改import tensorflow as tf from keras.models import Sequential from keras.layers import Dense, Dropout, Flatten from keras.layers import Conv2D, MaxPooling2D from keras.optimizers import SGD from keras.utils import np_utils from keras.preprocessing.image import ImageDataGenerator from keras.applications.vgg16 import VGG16 import numpy # 加载FER2013数据集 with open('E:/BaiduNetdiskDownload/fer2013.csv') as f: content = f.readlines() lines = numpy.array(content) num_of_instances = lines.size print("Number of instances: ", num_of_instances) # 定义X和Y X_train, y_train, X_test, y_test = [], [], [], [] # 按行分割数据 for i in range(1, num_of_instances): try: emotion, img, usage = lines[i].split(",") val = img.split(" ") pixels = numpy.array(val, 'float32') emotion = np_utils.to_categorical(emotion, 7) if 'Training' in usage: X_train.append(pixels) y_train.append(emotion) elif 'PublicTest' in usage: X_test.append(pixels) y_test.append(emotion) finally: print("", end="") # 转换成numpy数组 X_train = numpy.array(X_train, 'float32') y_train = numpy.array(y_train, 'float32') X_test = numpy.array(X_test, 'float32') y_test = numpy.array(y_test, 'float32') # 数据预处理 X_train /= 255 X_test /= 255 X_train = X_train.reshape(X_train.shape[0], 48, 48, 1) X_test = X_test.reshape(X_test.shape[0], 48, 48, 1) # 定义VGG16模型 vgg16_model = VGG16(weights='imagenet', include_top=False, input_shape=(48, 48, 3)) # 微调模型 model = Sequential() model.add(vgg16_model) model.add(Flatten()) model.add(Dense(256, activation='relu')) model.add(Dropout(0.5)) model.add(Dense(7, activation='softmax')) for layer in model.layers[:1]: layer.trainable = False # 定义优化器和损失函数 sgd = SGD(lr=0.01, decay=1e-6, momentum=0.9, nesterov=True) model.compile(optimizer=sgd, loss='categorical_crossentropy', metrics=['accuracy']) # 数据增强 datagen = ImageDataGenerator( featurewise_center=False, featurewise_std_normalization=False, rotation_range=20, width_shift_range=0.2, height_shift_range=0.2, horizontal_flip=True) datagen.fit(X_train) # 训练模型 model.fit_generator(datagen.flow(X_train, y_train, batch_size=32), steps_per_epoch=len(X_train) / 32, epochs=10) # 评估模型 score = model.evaluate(X_test, y_test, batch_size=32) print("Test Loss:", score[0]) print("Test Accuracy:", score[1])
这个错误是由于卷积层(Conv2D)中的过滤器深度与输入张量深度不匹配导致的。在这个例子中,过滤器深度(filter depth)是3,但是输入张量(input depth)的深度是1,无法整除,因此出现错误。为了解决这个问题,我们需要用一个输入深度能够被过滤器深度整除的模型输入数据。
代码改动可以通过如下方式完成:
1. 修改模型输入数据的维度,以匹配过滤器深度的要求。
2. 转换输入数据的颜色通道,以生成一个3个通道的输入张量。
下面是一个修改后的代码示例:
import tensorflow as tf
from keras.models import Sequential
from keras.layers import Dense, Dropout, Flatten
from keras.layers import Conv2D, MaxPooling2D
from keras.optimizers import SGD
from keras.utils import np_utils
from keras.preprocessing.image import ImageDataGenerator
from keras.applications.vgg16 import VGG16
import numpy as np
# 修改输入数据的维度
img_rows, img_cols = 32, 32
input_shape = (img_rows, img_cols, 3)
# 载入数据集
(x_train, y_train), (x_test, y_test) = tf.keras.datasets.cifar10.load_data()
# 将数据转换为浮点数类型
x_train = x_train.astype('float32')
x_test = x_test.astype('float32')
# 将像素值归一化到[0, 1]
x_train /= 255
x_test /= 255
# 将类向量转换为二进制类矩阵
num_classes = 10
y_train = np_utils.to_categorical(y_train, num_classes)
y_test = np_utils.to_categorical(y_test, num_classes)
# 生成并优化模型
model = Sequential()
model.add(Conv2D(32, (3, 3), activation='relu', input_shape=input_shape))
model.add(Conv2D(32, (3, 3), activation='relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Dropout(0.25))
model.add(Flatten())
model.add(Dense(128, activation='relu'))
model.add(Dropout(0.5))
model.add(Dense(num_classes, activation='softmax'))
sgd = SGD(lr=0.01, decay=1e-6, momentum=0.9, nesterov=True)
model.compile(loss='categorical_crossentropy',
optimizer=sgd,
metrics=['accuracy'])
# 在训练数据上生成扩增的数据
batch_size = 100
epochs = 5
datagen = ImageDataGenerator(
featurewise_center=False, # 将输入数据集按均值去中心化
samplewise_center=False, # 将每个样本按均值去中心化
featurewise_std_normalization=False, # 将输入数据除以数据集的标准差
samplewise_std_normalization=False, # 将每个样本除以自身的标准差
zca_whitening=False, # ZCA白化
rotation_range=0, # 随机旋转图像范围
width_shift_range=0.1, # 随机水平移动图像范围
height_shift_range=0.1, # 随机垂直移动图像范围
horizontal_flip=True, # 随机翻转图像
vertical_flip=False # # 随机翻转图像
)
datagen.fit(x_train)
model.fit(datagen.flow(x_train, y_train,
batch_size=batch_size),
epochs=epochs,
validation_data=(x_test, y_test),
steps_per_epoch=x_train.shape[0] // batch_size)
# 输出模型的准确率
scores = model.evaluate(x_test, y_test, verbose=1)
print('Test loss:', scores[0])
print('Test accuracy:', scores[1])
阅读全文
相关推荐
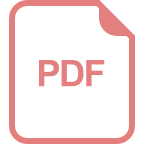
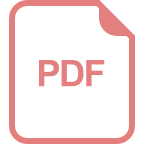
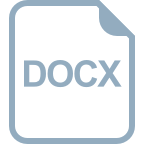
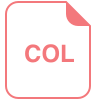
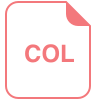
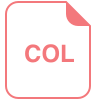
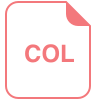
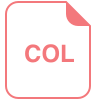
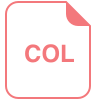
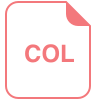
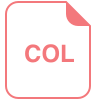
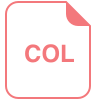
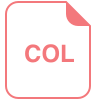
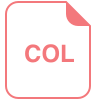
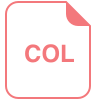
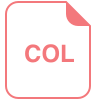