python rpyc 生产者消费者
时间: 2025-01-09 08:49:12 浏览: 3
### 使用 Python 和 RPyC 实现生产者消费者模式
在现代分布式应用开发中,生产者消费者模式是一种常见的方式用于解耦生产和消费逻辑。通过RPyC的服务导向编程模型可以很好地实现这一模式。
#### 创建服务端代码
首先创建一个名为 `ProducerConsumerServer.py` 的文件来定义生产者和消费者的RPC服务:
```python
import rpyc
from threading import Thread, Lock
from queue import Queue
class ProducerConsumerService(rpyc.Service):
def __init__(self):
super().__init__()
self.queue = Queue()
self.lock = Lock()
def exposed_put(self, item): # 生产者的接口方法
with self.lock:
print(f"Putting {item}")
self.queue.put(item)
def exposed_get(self): # 消费者的接口方法
while True:
try:
with self.lock:
value = self.queue.get(timeout=5)
print(f"Getting {value}")
return value
except Exception as e:
continue
if __name__ == "__main__":
from rpyc.utils.server import ThreadedServer
t = ThreadedServer(ProducerConsumerService(), port=18861)
print("Starting server...")
t.start() # 启动服务器[^2]
```
这段代码实现了基本的队列机制并暴露了两个远程可访问的方法给客户端使用:一个是放置新项目到共享缓冲区(`put`);另一个是从该区域取出下一个可用项(`get`)。
#### 客户端脚本编写
接着构建两个独立运行的程序分别模拟生产者行为以及消费者行为。这里假设两者都连接至同一台主机上的上述启动好的RPyC Server实例。
##### 生产者客户端 (`producer_client.py`)
此部分负责不断向指定地址发送消息作为输入数据流的一部分:
```python
import time
import random
import rpyc
conn = rpyc.connect('localhost', 18861) # 建立与服务端的连接
try:
for i in range(10):
message = f'Message-{i}'
conn.root.put(message) # 调用远端提供的 put 方法
sleep_time = random.uniform(.5, 3.)
time.sleep(sleep_time)
finally:
conn.close() # 关闭连接
```
##### 消费者客户端 (`consumer_client.py`)
这部分则持续尝试获取来自相同位置的消息直到遇到异常情况停止工作:
```python
import time
import rpyc
conn = rpyc.connect('localhost', 18861) # 建立与服务端的连接
while True:
try:
received_message = conn.root.get() # 获取远端 get 返回的结果
print(f'Consuming: {received_message}')
time.sleep(random.random()) # 处理接收到的数据所需的时间
except EOFError:
break
finally:
conn.close() # 断开连接
```
以上即为利用Python结合RPyC框架搭建简易版本生产者-消费者系统的具体实践案例。值得注意的是,在实际应用场景下还需要考虑更多因素如错误处理、性能优化等方面的问题。
阅读全文
相关推荐







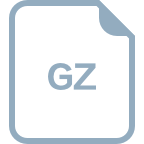









