public static void copyFolder(File sourceFolder, File destFolder) throws IOException { if (!destFolder.exists()) { destFolder.mkdir(); } File[] files = sourceFolder.listFiles(); for (File file : files) { if (file.isDirectory()) { File newFolder = new File(destFolder, file.getName()); copyFolder(file, newFolder); } else { File newFile = new File(destFolder, file.getName()); copyFile(file, newFile); } } } public static void copyFile(File sourceFile, File destFile) throws IOException { try (FileInputStream fis = new FileInputStream(sourceFile); FileOutputStream fos = new FileOutputStream(destFile); FileChannel sourceChannel = fis.getChannel(); FileChannel destChannel = fos.getChannel()) { destChannel.transferFrom(sourceChannel, 0, sourceChannel.size()); } }
时间: 2024-02-15 20:27:46 浏览: 104
这段代码可以用于复制一个文件夹及其中的所有文件和子文件夹到另一个目录。它会首先创建目标文件夹,然后遍历源文件夹中的所有文件和子文件夹,对于每个子文件夹,它会递归调用copyFolder方法进行复制,对于每个文件,它会调用copyFile方法进行复制。
copyFile方法使用了Java NIO的FileChannel类,其中transferFrom方法可以将一个通道的数据传输到另一个通道中。这里使用了try-with-resources语句,确保在方法执行完成后自动关闭所有打开的资源。
需要注意的是,这段代码只会复制文件夹中的文件和子文件夹,并不会复制文件夹本身的属性和元数据。如果需要完全复制一个文件夹,需要使用更复杂的方法。
阅读全文
相关推荐
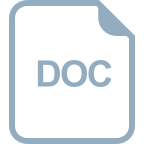
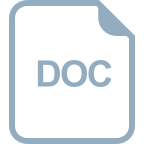
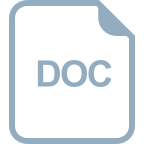
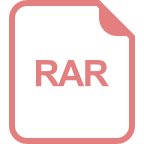
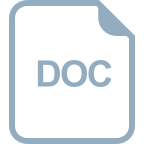
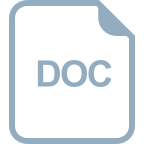
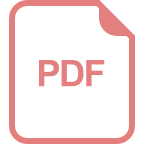
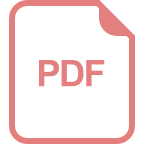
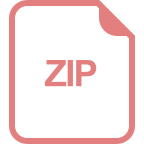
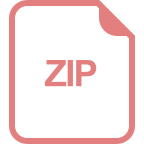