帮我用C语言编程: 输入10个学生5门课成绩,分别用函数求: 1)每个学生平均分; 2)每门课的平均分; 3)找出最高的分数所对应的学生和课程;
时间: 2024-05-04 21:17:52 浏览: 14
好的,以下是用 C 语言编写的程序,实现输入 10 个学生 5 门课的成绩,并求解每个学生的平均分、每门课的平均分以及找出最高分数所对应的学生和课程:
```c
#include <stdio.h>
#define NUM_STUDENTS 10
#define NUM_COURSES 5
void calcAvgScorePerStudent(float scores[NUM_STUDENTS][NUM_COURSES], float avgScores[NUM_STUDENTS]) {
int i, j;
for (i = 0; i < NUM_STUDENTS; i++) {
float sum = 0;
for (j = 0; j < NUM_COURSES; j++) {
sum += scores[i][j];
}
avgScores[i] = sum / NUM_COURSES;
}
}
void calcAvgScorePerCourse(float scores[NUM_STUDENTS][NUM_COURSES], float avgScores[NUM_COURSES]) {
int i, j;
for (j = 0; j < NUM_COURSES; j++) {
float sum = 0;
for (i = 0; i < NUM_STUDENTS; i++) {
sum += scores[i][j];
}
avgScores[j] = sum / NUM_STUDENTS;
}
}
void findHighestScore(float scores[NUM_STUDENTS][NUM_COURSES], int *studentIdx, int *courseIdx) {
int i, j;
float highestScore = -1;
for (i = 0; i < NUM_STUDENTS; i++) {
for (j = 0; j < NUM_COURSES; j++) {
if (scores[i][j] > highestScore) {
highestScore = scores[i][j];
*studentIdx = i;
*courseIdx = j;
}
}
}
}
int main() {
float scores[NUM_STUDENTS][NUM_COURSES];
float avgScoresPerStudent[NUM_STUDENTS] = {0};
float avgScoresPerCourse[NUM_COURSES] = {0};
int highestScoreStudentIdx, highestScoreCourseIdx;
int i, j;
// 输入成绩
printf("请输入 10 个学生 5 门课的成绩:\n");
for (i = 0; i < NUM_STUDENTS; i++) {
printf("学生 %d:", i + 1);
for (j = 0; j < NUM_COURSES; j++) {
scanf("%f", &scores[i][j]);
}
}
// 计算每个学生的平均分
calcAvgScorePerStudent(scores, avgScoresPerStudent);
printf("\n每个学生的平均分:");
for (i = 0; i < NUM_STUDENTS; i++) {
printf("%.2f ", avgScoresPerStudent[i]);
}
// 计算每门课的平均分
calcAvgScorePerCourse(scores, avgScoresPerCourse);
printf("\n每门课的平均分:");
for (j = 0; j < NUM_COURSES; j++) {
printf("%.2f ", avgScoresPerCourse[j]);
}
// 找出最高分数所对应的学生和课程
findHighestScore(scores, &highestScoreStudentIdx, &highestScoreCourseIdx);
printf("\n最高分数为 %.2f,对应的学生为 %d,课程为 %d\n", scores[highestScoreStudentIdx][highestScoreCourseIdx], highestScoreStudentIdx + 1, highestScoreCourseIdx + 1);
return 0;
}
```
程序中使用了三个函数:
- `calcAvgScorePerStudent`:计算每个学生的平均分,将结果保存在 `avgScores` 数组中。
- `calcAvgScorePerCourse`:计算每门课的平均分,将结果保存在 `avgScores` 数组中。
- `findHighestScore`:找出最高分数所对应的学生和课程,将结果保存在 `studentIdx` 和 `courseIdx` 变量中。
在 `main` 函数中,首先输入成绩,然后分别调用上述三个函数,输出每个学生的平均分、每门课的平均分以及最高分数所对应的学生和课程。
相关推荐
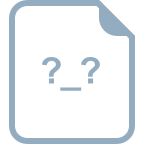
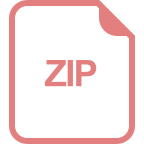
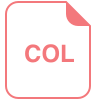
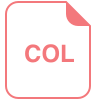
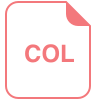
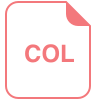
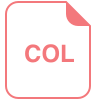









