python编写小游戏cfg
时间: 2023-07-17 17:09:37 浏览: 217
当然可以!下面是一个示例的 Python 代码,实现了一个简单的文本冒险游戏:
```python
def start_game():
print("欢迎来到冒险游戏!")
print("你发现自己在一个神秘的森林中。")
print("你的目标是找到宝藏并成功逃离这个森林。")
print("你可以选择向前走、向左走或向右走。")
while True:
choice = input("请选择你的下一步(前/左/右):")
if choice == "前":
print("你向前走了一段距离。")
# 在这里可以编写更多的游戏逻辑
elif choice == "左":
print("你向左转了。")
# 在这里可以编写更多的游戏逻辑
elif choice == "右":
print("你向右转了。")
# 在这里可以编写更多的游戏逻辑
else:
print("无效的选择,请重新选择。")
start_game()
```
这只是一个简单的示例,你可以根据自己的需求扩展代码,加入更多的游戏逻辑和场景描述。希望对你有所帮助!
相关问题
python编写飞机大战小游戏cfg
您好!以下是一个简单的飞机大战小游戏的 Python 代码示例:
```python
import pygame
import random
# 初始化游戏
pygame.init()
# 游戏窗口大小
screen_width = 480
screen_height = 600
# 创建游戏窗口
screen = pygame.display.set_mode((screen_width, screen_height))
# 设置窗口标题
pygame.display.set_caption("飞机大战")
# 加载背景图片
background = pygame.image.load("background.png")
# 加载玩家飞机图片
player = pygame.image.load("player.png")
player_rect = player.get_rect()
player_rect.centerx = screen_width // 2
player_rect.bottom = screen_height - 10
# 加载敌机图片
enemy = pygame.image.load("enemy.png")
enemy_rect = enemy.get_rect()
# 计时器,用于控制敌机的出现频率
enemy_timer = pygame.time.get_ticks()
# 子弹的初始速度
bullet_speed = 5
# 子弹列表
bullets = []
# 敌机列表
enemies = []
# 游戏结束标志位
game_over = False
# 游戏时钟
clock = pygame.time.Clock()
# 游戏循环
while not game_over:
# 设置帧率
clock.tick(60)
# 监听事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
# 监听键盘事件,控制玩家飞机移动和发射子弹
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_SPACE:
bullet = pygame.Rect(player_rect.centerx, player_rect.top, 5, 11)
bullets.append(bullet)
# 移动玩家飞机
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT]:
player_rect.x -= 5
if keys[pygame.K_RIGHT]:
player_rect.x += 5
if keys[pygame.K_UP]:
player_rect.y -= 5
if keys[pygame.K_DOWN]:
player_rect.y += 5
# 控制玩家飞机在窗口范围内移动
if player_rect.left < 0:
player_rect.left = 0
if player_rect.right > screen_width:
player_rect.right = screen_width
if player_rect.top < 0:
player_rect.top = 0
if player_rect.bottom > screen_height:
player_rect.bottom = screen_height
# 发射子弹
for bullet in bullets:
bullet.y -= bullet_speed
if bullet.y < 0:
bullets.remove(bullet)
# 控制敌机的出现频率
now = pygame.time.get_ticks()
if now - enemy_timer >= 1000:
enemy_rect.x = random.randint(0, screen_width - enemy_rect.width)
enemy_rect.y = -enemy_rect.height
enemies.append(enemy_rect)
enemy_timer = now
# 移动敌机
for enemy in enemies:
enemy.y += bullet_speed
if enemy.y > screen_height:
enemies.remove(enemy)
# 碰撞检测
for bullet in bullets:
for enemy in enemies:
if bullet.colliderect(enemy):
bullets.remove(bullet)
enemies.remove(enemy)
# 绘制背景
screen.blit(background, (0, 0))
# 绘制玩家飞机
screen.blit(player, player_rect)
# 绘制子弹
for bullet in bullets:
pygame.draw.rect(screen, (255, 255, 255), bullet)
# 绘制敌机
for enemy in enemies:
screen.blit(enemy, enemy)
# 更新屏幕
pygame.display.update()
# 退出游戏
pygame.quit()
```
请注意,以上代码只是一个简单的示例,您可以根据自己的需求进行修改和完善。同时,您需要准备好相应的图片资源,并将其与代码放在同一个目录下。希望对您有所帮助!
python小游戏源代码无cfg
Python小游戏源代码没有cfg文件,其实是完全可以的。cfg文件通常用于存储配置信息,例如游戏设置、用户偏好等。但是,并不是每个Python小游戏都需要使用cfg文件来存储配置信息。
在编写Python小游戏源代码时,可以选择将配置信息直接写在代码中。例如,你可以使用变量来存储游戏设置,如游戏背景颜色、游戏速度等。这样做的好处是,代码更加简洁,可读性也更高。
另一种方法是使用字典来存储配置信息。字典是一种可变容器,可以通过键-值对的形式存储数据。你可以将游戏的各种配置信息存储在一个字典中,然后在代码中通过键来访问相应的值。
此外,你还可以将配置信息存储在一个独立的.py文件中,然后在主游戏代码中导入该文件。这样可以将配置信息和主代码进行分离,使得代码更具可维护性。
总之,Python小游戏源代码并不一定需要cfg文件来存储配置信息。你可以选择将配置信息直接写在代码中,使用字典存储配置信息,或者将配置信息存储在单独的文件中,以方便维护和修改。
阅读全文
相关推荐
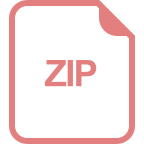
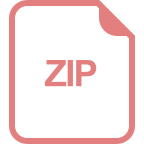
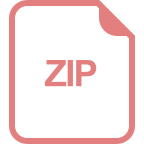
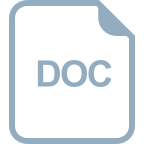

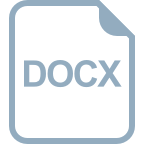
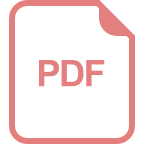
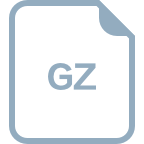
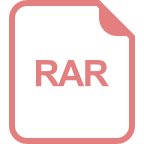
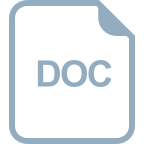
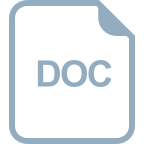
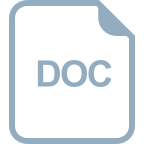
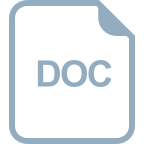
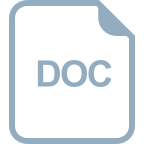
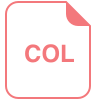